Table of Contents
Why Flexible Logical Thinking Matters for Developers
Rust MUD Game is one of the best ways to practice flexible logical thinking and structure a real-world application efficiently.
In the world of software development, flexible logical thinking isn’t just helpful — it’s essential.
No matter which language you use — Rust, C, Python, Java, or even JavaScript — the core of programming remains the same:
You must structure your thoughts clearly, and translate them into working logic.
Real-world programming rarely gives you a perfectly clear set of instructions.
You’ll face:
- Ambiguous requirements
- Changing user needs
- Unexpected errors
In these cases, developers who can adapt their logic freely survive and thrive.
Rigid, textbook thinking is not enough.
We must practice building our own dynamic logic systems, starting from small, manageable projects.
If you haven’t set up Rust yet, check out our guide on How to Set Up a Rust Development Environment on Windows.
Why All Programming Languages Require This Skill
Programming languages are different only on the surface.
You can learn more about Rust itself by visiting the official Rust website.
Language | Syntax | Core Logic |
---|---|---|
Rust | Strong typing, safety first | Structuring logic |
C | Manual memory management | Structuring logic |
Python | Easy syntax | Structuring logic |
Java | Object-oriented | Structuring logic |
JavaScript | Asynchronous programming | Structuring logic |
✅ No matter which tool you pick,
✅ No matter how beautiful the syntax is,
👉 The real game is about how well you control logical flow.
By mastering it here, with a simple Rust MUD Game,
you’ll unlock your ability to adapt to any language later.
Today’s Focus: Building the Map System
We’re not trying to build a complete game today.
We have a narrow but critical focus: The Map.
Why start with the map?
- Movement and navigation are the core of any game world.
- Handling movement trains you in state management.
- Designing connected rooms trains you in graph-like structures, essential in real-world apps.
✅ If you can build a flexible, expandable map system,
adding monsters, battles, and items later becomes natural.
Logical Flowchart (Detailed)
Here’s how the Rust MUD Game flow looks, expanded with full logical steps:
[Start Game]
↓
[Set Player Starting Location]
↓
[Show Current Room Name & Description]
↓
[Prompt Player for Direction Input]
↓
[Match Input Command]
↓
Valid Direction? (north/south/east/west)
├── Yes → [Move Player to Next Room]
│ ↓
│ [Loop Back: Show New Room]
└── No → [Show Error Message]
↓
[Ask Again]
↓
If Input is "quit" → [Exit Game Gracefully]
✅ Every step matches exactly what we will write in code.
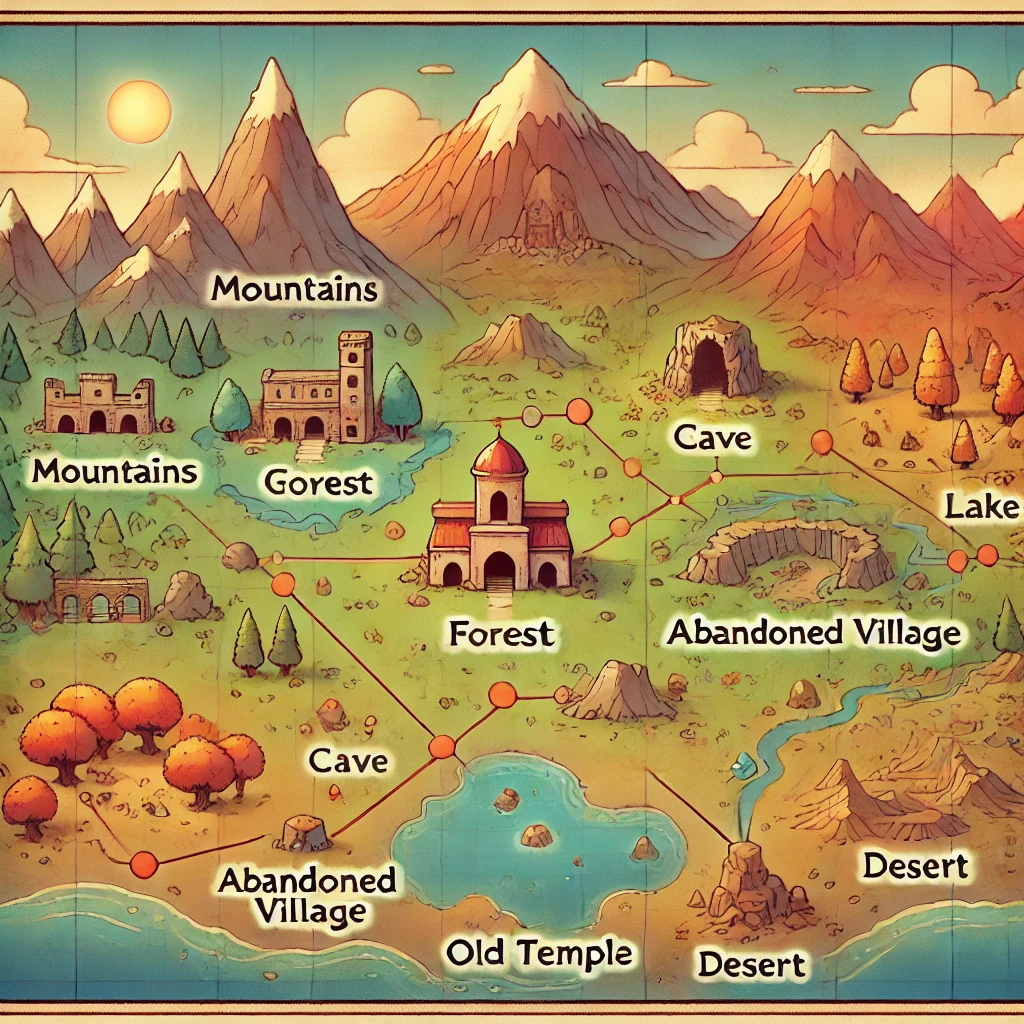
Full Rust Code (With Detailed Comments and Logical Flow Matching)
// Import required libraries
use std::collections::HashMap; // For managing rooms efficiently
use std::io; // For handling user input
// Step 1: Define Room structure
struct Room {
name: &'static str, // Room's display name
description: &'static str, // Text shown when the player enters
north: Option<&'static str>, // Name of room to the north (if any)
south: Option<&'static str>, // Name of room to the south (if any)
east: Option<&'static str>, // Name of room to the east (if any)
west: Option<&'static str>, // Name of room to the west (if any)
}
fn main() {
// Step 2: Setup the map (the world)
let mut rooms = HashMap::new();
// Insert rooms into the map
rooms.insert("Mountains", Room {
name: "Mountains",
description: "You are high in the rocky mountains.",
north: None,
south: Some("Forest"),
east: None,
west: None,
});
rooms.insert("Forest", Room {
name: "Forest",
description: "You are standing in a dense, dark forest.",
north: Some("Mountains"),
south: Some("Abandoned Village"),
east: Some("Cave"),
west: None,
});
rooms.insert("Cave", Room {
name: "Cave",
description: "You are inside a damp cave.",
north: None,
south: None,
east: Some("Lake"),
west: Some("Forest"),
});
rooms.insert("Lake", Room {
name: "Lake",
description: "You stand by a clear, blue lake.",
north: None,
south: None,
east: None,
west: Some("Cave"),
});
rooms.insert("Abandoned Village", Room {
name: "Abandoned Village",
description: "You are in an abandoned, silent village.",
north: Some("Forest"),
south: Some("Old Temple"),
east: None,
west: None,
});
rooms.insert("Old Temple", Room {
name: "Old Temple",
description: "You are in the ruins of an ancient temple.",
north: Some("Abandoned Village"),
south: None,
east: Some("Desert"),
west: None,
});
rooms.insert("Desert", Room {
name: "Desert",
description: "You wander a vast, hot desert.",
north: None,
south: None,
east: None,
west: Some("Old Temple"),
});
// Step 3: Initialize the player
let mut current_location = "Forest"; // Start in the Forest
println!("🏕️ Welcome to the Rust MUD Game!");
println!("Type 'north', 'south', 'east', 'west' to move, or 'quit' to exit.");
// Step 4: Main Game Loop
loop {
// Show current room
let room = rooms.get(current_location).unwrap();
println!("\n📍 Location: {}", room.name);
println!("{}", room.description);
// Ask for direction input
println!("\nWhich direction do you want to go?");
let mut direction = String::new();
io::stdin().read_line(&mut direction).expect("Failed to read input");
// Match user input
match direction.trim() {
"north" => {
if let Some(next_room) = room.north {
current_location = next_room;
} else {
println!("🚫 You can't go north from here.");
}
}
"south" => {
if let Some(next_room) = room.south {
current_location = next_room;
} else {
println!("🚫 You can't go south from here.");
}
}
"east" => {
if let Some(next_room) = room.east {
current_location = next_room;
} else {
println!("🚫 You can't go east from here.");
}
}
"west" => {
if let Some(next_room) = room.west {
current_location = next_room;
} else {
println!("🚫 You can't go west from here.");
}
}
"quit" => {
println!("👋 Thanks for playing! Goodbye!");
break; // Exit the game loop
}
_ => {
println!("❓ Invalid command. Please type 'north', 'south', 'east', 'west', or 'quit'.");
}
}
}
}
Practical Application of This MUD Map Logic
area | applicability |
---|---|
Text-Based Adventure Games | Core movement and exploration systems |
Web Application Routing | Moving between pages or states |
Game Server State Management | Handling player locations on server |
Graph Data Structures | Network, graph algorithm simulations |
✅ Simple today, but extremely powerful in real applications!
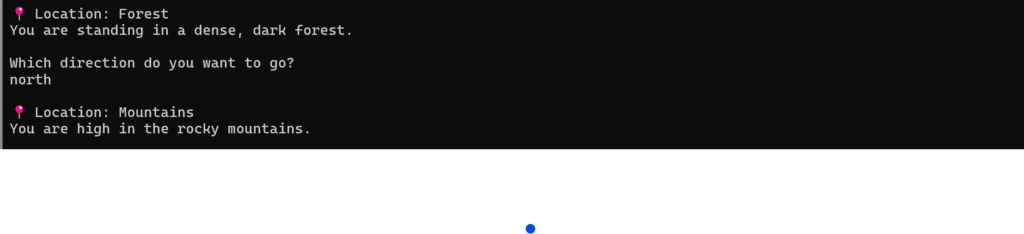
Conclusion: Shape the World with Your Code
This Rust MUD Game map system may seem simple at first glance,
but within its modest structure lies a powerful truth:
You hold the power to shape worlds with your code.
Every room you define,
every path you connect,
every small decision you make in your logic
is an act of creation.
It is not just about moving a player north, south, east, or west.
It is about learning to control possibilities,
building freedom from structure,
turning ideas into living, breathing systems.
Think of each room as a seed,
and your imagination as the soil.
With every new feature you imagine —
whether it’s a secret passage, a hidden monster, or a mysterious event —
you are planting the seeds of your own universe.
You are not just learning Rust.
You are learning how to think like a creator.
- Break apart the limitations placed on you.
- Expand your vision beyond simple tutorials.
- Breathe life into empty spaces with nothing but your logic and creativity.
The screen in front of you is not just a canvas —
it is the raw material of a universe waiting for your hand to shape it.
If you can freely master this tiny map system,
you will soon find yourself comfortable building:
- Full-fledged games,
- Intelligent server systems,
- Dynamic simulations,
- Even entire living worlds.
Because what matters is not the complexity of the tools,
but the freedom of the mind that wields them.
🌍 Take ownership of this project.
🎨 Make the Rust MUD Game your own masterpiece.
🚀 Build the world you’ve always imagined — room by room, idea by idea, line by line.
You have everything you need right now:
A keyboard.
A mind that dares to create.
And a world waiting to be born.
Stay tuned for Part 2,
where we’ll ignite the world with random monster encounters,
and your map will begin to pulse with life and adventure.
This is just the beginning.
Your story as a creator starts here.