(Made by Me, Designed for Ultimate Logical Flow Mastery)
Before We Start
If you have not set up Rust development environment on Windows yet,
👉 Please read this guide first:
How to Set Up Rust Development Environment on WindowsThis project assumes you already have Rust and Cargo installed!
Table of Contents
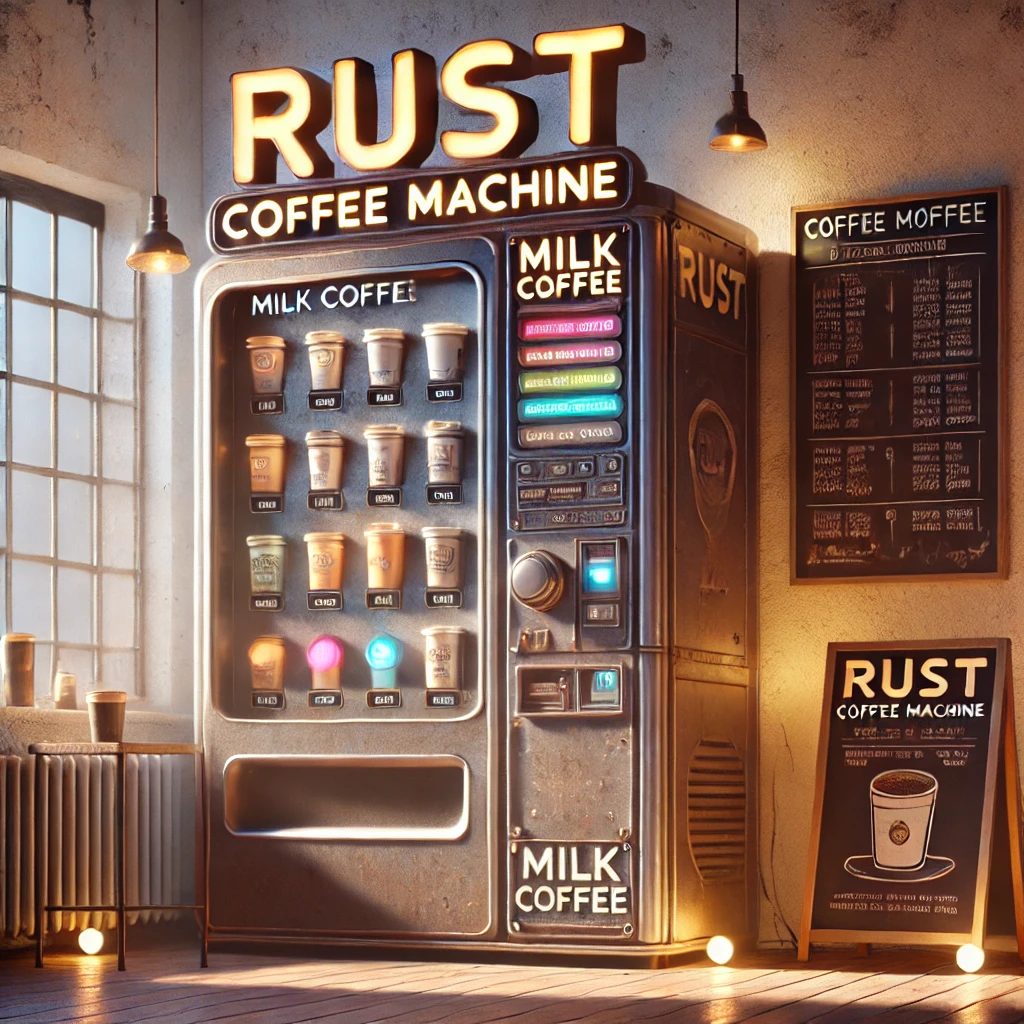
Introduction
Welcome! ☕
Today I want to proudly introduce a project that I personally designed and built:
The Rust Coffee Vending Machine Simulator.
Why did I create this?
Because mastering logical flow is the secret key to becoming a real developer.
Without strong logical thinking, no amount of fancy libraries or GUIs can help.
And trust me — this small but complete project will teach you how to think step-by-step like a real computer!
✅ You will practice:
- Making decisions
- Handling user input safely
- Managing inventory like a real machine
- Simulating real-world processes logically
Important: Install rand
Library First!
Before you even start coding,
👉 You MUST add the rand
library.
Why?
Because we will simulate random temperatures (like a real heating machine),
and Rust does not include random number generation by default to keep it lightweight.
If you forget to add it, your code will throw errors like:
“unresolved import
rand
“
“cannot find functionthread_rng
in craterand
“
👉 To install it, open your terminal inside the project folder and type:
cargo add rand
✅ This installs the latest rand 0.9 version,
which introduces the new, easier rand::rng()
and random_range()
functions.
Without this step, the simulator WILL NOT COMPILE!
Why This Project Is the Best for Learning Logical Flow
이유 | 설명 |
---|---|
Real-World Simulation | Heating, menu choice, payment, and stock management — just like real machines! |
Clear Step-by-Step Thinking | You control everything: input → decision → reaction |
Transferable to Other Languages (like C) | Logical thinking is universal: once you master it here, you can apply it in C, C++, Python, Java and everywhere! |
Fast Skill Growth | Practicing small but real scenarios sharpens your mind faster than just copying tutorials |
This is the secret:
“Mastering logical flow here makes any future coding project much easier.”
Logical Flowchart
[Start]
↓
[Check if Coffee Exists]
↓
[Heat Machine to 80°C+]
↓
[Show Drink Menu]
↓
[User Selects Drink]
↓
[User Sets Sugar Level]
↓
[Insert 25¢ Coin]
↓
[Check Ingredient Availability]
↓
(Enough?)
├── Yes → [Brew Coffee → Update Inventory → Show Remaining Stock → Check if Stock is Empty]
└── No → [Refund Coin → End]
↓
[End]
✅ This flow teaches clear problem-solving thinking.
Full Rust Code (Friendly Comments, rand 0.9 Version)
// rand 0.9 version is required!
// Import random number generator
use rand::Rng;
// Import libraries for input, sleep, and time control
use std::{io, thread, time};
fn main() {
// Set initial inventory (in grams)
let mut sugar = 500;
let mut creamer = 400;
let mut coffee = 600;
// Define how much each spoon uses
let sugar_per_spoon = 5; // 5 grams per spoon of sugar
let creamer_per_spoon = 4; // 4 grams per spoon of creamer
let coffee_per_spoon = 6; // 6 grams per spoon of coffee
println!("☕ Welcome to the Rust Coffee Vending Machine Simulator!");
// Step 1: Check if any coffee exists
if coffee == 0 {
println!("Sorry, no coffee left. Machine shutting down.");
return;
}
// Step 2: Heat the machine
loop {
let mut rng = rand::rng(); // create a random number generator
let temperature = rng.random_range(50..=100); // pick a number between 50 and 100
println!("Current Temperature: {}°C", temperature);
if temperature >= 80 {
println!("Temperature is good! Ready to serve!");
break; // Exit the loop
} else {
println!("Heating... Please wait 2 seconds.");
thread::sleep(time::Duration::from_secs(2)); // Wait 2 seconds
}
}
// Step 3: Show the drink menu
let drink_type = loop {
println!("=========================");
println!(" Menu");
println!("=========================");
if creamer > 0 {
println!("1. Milk Coffee (with creamer)");
}
println!("2. Black Coffee (no creamer)");
println!("=========================");
println!("Select your drink:");
let mut menu_input = String::new();
io::stdin().read_line(&mut menu_input).expect("Failed to read input");
let menu_choice: u32 = match menu_input.trim().parse() {
Ok(num) => num,
Err(_) => {
println!("Please enter a valid number.");
continue; // Try again
}
};
match menu_choice {
1 if creamer > 0 => {
println!("You chose Milk Coffee!");
break 1;
}
2 => {
println!("You chose Black Coffee!");
break 2;
}
_ => {
println!("Invalid choice. Please try again.");
}
}
};
// Step 4: Ask how many spoons of sugar
println!("How many spoons of sugar would you like? (0 to 5):");
let sugar_spoons = read_number();
// Step 5: Insert one 25¢ coin
println!("Please insert one 25¢ coin:");
let coin = read_number();
if coin != 25 {
println!("Only one 25¢ coin is accepted. Canceling order.");
return;
}
println!("Payment received! Preparing your coffee...");
// Step 6: Calculate the needed ingredients
let (sugar_needed, creamer_needed, coffee_needed) = match drink_type {
1 => (sugar_spoons * sugar_per_spoon, 4 * creamer_per_spoon, 3 * coffee_per_spoon),
2 => (sugar_spoons * sugar_per_spoon, 0, 5 * coffee_per_spoon),
_ => (0, 0, 0),
};
// Step 7: Check if enough ingredients
if sugar < sugar_needed || creamer < creamer_needed || coffee < coffee_needed {
println!("Sorry, not enough ingredients. Refunding your 25¢.");
return;
}
// Step 8: Brew the coffee
println!("Brewing your coffee... Please wait!");
thread::sleep(time::Duration::from_secs(3));
println!("✅ Your coffee is ready! Enjoy!");
// Step 9: Subtract used ingredients
sugar -= sugar_needed;
creamer -= creamer_needed;
coffee -= coffee_needed;
// Step 10: Show remaining stock
println!("---------------------------");
println!("Remaining Ingredients:");
println!("Sugar: {} grams", sugar);
println!("Creamer: {} grams", creamer);
println!("Coffee: {} grams", coffee);
println!("---------------------------");
// Step 11: Check if coffee is finished
if coffee == 0 {
println!("⚠️ Coffee stock depleted. Machine shutting down.");
}
}
// Helper function to read numbers safely
fn read_number() -> u32 {
let mut input = String::new();
io::stdin().read_line(&mut input).expect("Failed to read input");
input.trim().parse().unwrap_or(0) // Return 0 if failed
}
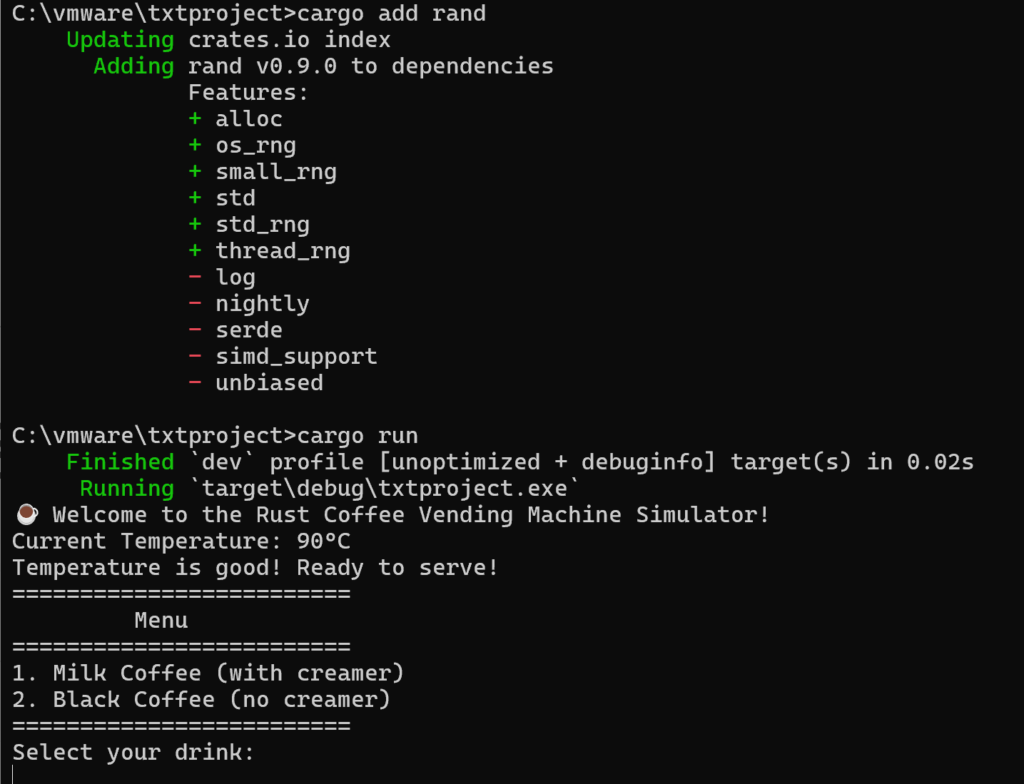
Conclusion
✅ By building this Coffee Vending Machine, you learned:
- How to think like a machine (step-by-step!)
- How to handle input and errors cleanly
- How to simulate real processes easily
- How to use Rust’s random tools safely (rand 0.9)
Logical flow mastery first. Then anything becomes easy.
I’m proud of this project because it’s a real shortcut to smart programming. 🚀
And remember:
This logical approach is NOT just for Rust.
✅ It is also powerful in C, C++, Java, Python — ANY language!
External Resources
If you want to learn more about Rust, here are some trusted sources:
- Rust – Wikipedia
→ A comprehensive overview of Rust, including its history, features, and design goals. - The Rust Programming Language – Official Website
→ The official Rust site for downloads, documentation, and tutorials.