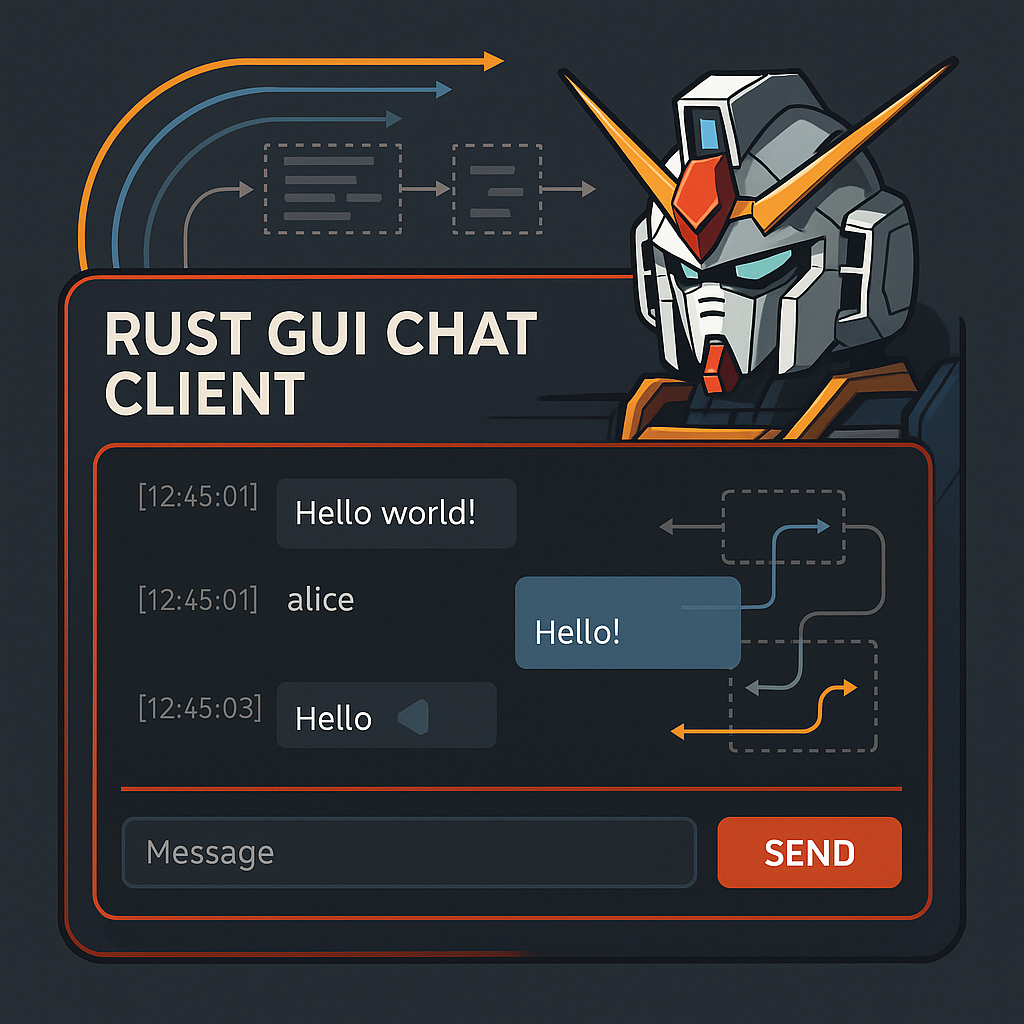
❓ What Is a Rust GUI Chat Client and Why Build One?
A Rust GUI chat client is a desktop chat application built with Rust and a graphical user interface framework like eGUI, designed for real-time communication over a network.
While many chat applications are built with scripting languages or web technologies, Rust offers an exceptional blend of speed, memory safety, and concurrency, making it ideal for building robust and responsive desktop applications.
🧱 What Makes It Unique?
Unlike terminal-based or web chat clients, a Rust GUI chat client combines:
- ✅ Performance — Rust compiles to native machine code, making the GUI smooth and responsive.
- ✅ Safety — Thanks to Rust’s strong type system and ownership model, the app avoids crashes and memory leaks.
- ✅ Asynchronous Networking — Rust’s async runtime () allows smooth real-time message handling without freezing the UI.
tokio
- ✅ Modern UX — Using , you can create an intuitive interface with text fields, scroll areas, and buttons—no HTML/CSS required.
egui
🎯 Why Should You Build One?
- 🚀 Hands-On Learning: Combining GUI + networking + async is an excellent real-world Rust project.
- 🧠 Concept Mastery: You’ll learn about TCP communication, message parsing, multithreading, and state management.
- 🛠 Portfolio-Ready: A functional desktop chat app is an impressive project for your GitHub or résumé.
- 🌍 Cross-platform Ready: Rust apps built with / run on Windows, macOS, and Linux.
eframe
egui
In short, building a Rust GUI chat client helps you master system-level performance with application-level usability.
Table of Contents
❓ How Does eGUI Make Rust GUI Chat Client Development Easier?
eGUI is a modern, immediate-mode GUI library for Rust that dramatically simplifies the development of desktop applications like a Rust GUI chat client.
It eliminates the need for complex GUI frameworks or foreign function interfaces, making it easier for Rust developers—especially beginners—to create rich, interactive, and cross-platform user interfaces with minimal code.
If you’ve ever tried building a GUI in C++ with Qt, or in Rust with GTK bindings, you know how steep the learning curve can be. In contrast, eGUI offers a pure-Rust solution with zero setup beyond adding a dependency. You write your UI layout in simple declarative blocks, and eGUI automatically handles the rendering each frame.
🧩 Key Features That Make eGUI Ideal for Rust GUI Chat Clients
Feature | Why It’s Perfect for Chat Client Apps |
---|---|
Immediate-mode architecture | Perfect for fast-changing interfaces like chat windows—UI state updates every frame |
Text input widgets | Built-in text fields for message input, nicknames, or settings |
Scrollable containers | Essential for viewing chat history or user lists |
Minimal setup | Just import eframe and you’re ready to build a full GUI window |
Lightweight and fast | No runtime dependencies, no need for linking native libraries |
Cross-platform | Runs on Windows, Linux, macOS with a single codebase |
WASM compatible | Can eventually turn your desktop app into a web-based client |
Custom painting & theming | Make your chat interface stylish with colors, rounded boxes, and emoji support |
🧪 How eGUI Handles Real-Time Messaging in Chat Clients
Real-time chat applications require responsive UI updates and background networking, often handled asynchronously. eGUI doesn’t block your app while you wait for user input or network responses. When integrated with Rust’s async features or message-passing systems (like tokio::sync::mpsc
), you can build a truly real-time chat interface that feels smooth and modern.
Each frame in eGUI can:
- Pull new messages from a shared buffer
- Display them instantly in a scrollable panel
- Respond to user input with zero noticeable lag
This frame-by-frame UI refresh model means that your chat interface stays in sync with the backend without managing complex state transitions manually.
🎯 Why Use eGUI Instead of Other Rust GUI Frameworks?
Other GUI libraries like gtk-rs
, druid
, or fltk-rs
are capable, but:
- ❌ They rely on C/C++ native bindings, which may introduce build errors or platform-specific bugs
- ❌ Their event-based model is harder to reason about for beginners
- ❌ They often require additional setup or external packages to run
eGUI, by comparison, is:
- ✅ 100% written in Rust
- ✅ Easy to learn and deploy
- ✅ Backed by a growing community and active development
- ✅ The foundation of
eframe
, a fully integrated app framework
💡 Developer Insight
Building a GUI chat client in Rust used to be hard—until eGUI came along. It brings the ease of immediate-mode design (like Dear ImGui) to Rust, while staying safe, fast, and elegant.
If you’re building a chat application where speed and responsiveness matter, eGUI is one of the most beginner-friendly yet powerful choices available in the Rust ecosystem today.
❓ What Tools Do You Need to Start Your Rust GUI Chat Client Project?
To build a Rust GUI chat client, you’ll need a combination of core development tools, libraries, and async networking frameworks that work together to provide real-time messaging and a modern desktop interface.
Even if you’re new to GUI or network programming, Rust’s toolchain and ecosystem offer a clean, manageable path. Let’s break down everything you need—from setting up Rust itself, to integrating GUI and async components into one seamless chat experience.
🧰 1. Rust Toolchain (cargo, rustc, rustup)
The foundation of every Rust project starts here:
rustup
: The recommended installer and version manager for Rust. It ensures you always have the latest stable version.cargo
: Rust’s package manager and build tool. You’ll use this to build your chat client, manage dependencies likeegui
, and run your project.rustc
: The Rust compiler itself. Normally used under the hood viacargo
.
🛠️ Install with:
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
📌 After installation, check with:
cargo --version
rustc --version
🎨 2. eGUI and eframe for the GUI Layer
Your Rust GUI chat client will need an interface—buttons, text inputs, chat bubbles, scrollable panels—and that’s where egui
and eframe
come in.
egui
: The lightweight immediate-mode GUI library that powers your interface.eframe
: A high-level wrapper aroundegui
that handles window creation and rendering—perfect for desktop apps.
🧱 Add these to your Cargo.toml
:
eframe = "0.27"
egui = "0.27"
You’ll use this to:
- Draw the chat window
- Add a text input for messages
- Show a scrollable list of chat history
- Add a “Send” button
🌐 3. Tokio: The Async Runtime Engine
Since your chat client communicates over a network, you’ll need asynchronous I/O to keep your interface responsive while waiting for messages from the server.
tokio
: Rust’s most popular async runtime. It enables you to open TCP connections, spawn async tasks, and manage real-time networking without blocking your GUI.
🧵 In Cargo.toml
:
tokio = { version = "1", features = ["full"] }
This will allow you to:
- Connect to a chat server (
TcpStream::connect
) - Read messages asynchronously (
BufReader::new(stream).lines()
) - Write messages (
stream.write_all(...)
) - Spawn background network threads
🔄 4. Message Channels (mpsc)
In a real-world chat client, GUI rendering and networking logic should run in separate threads. To pass data between them, we use channels:
tokio::sync::mpsc
: Allows GUI events (like typing or clicking “Send”) to be forwarded to a background networking task.
Example use:
let (tx, mut rx) = tokio::sync::mpsc::unbounded_channel();
This lets you:
- Write messages from the GUI and send them to the TCP connection thread
- Receive network messages and push them into your visible message list
🧠 5. Optional but Recommended
Tool | Why it’s helpful |
---|---|
anyhow | For easy error handling throughout your project |
log + env_logger | For debugging, especially in async code |
serde_json | To encode/decode structured chat messages (optional for advanced features) |
chrono | To add timestamps to chat messages |
wasm-bindgen | For building a WebAssembly version later (if desired) |
✅ Full Cargo.toml
Dependency Example
[dependencies]
tokio = { version = "1", features = ["full"] }
eframe = "0.27"
egui = "0.27"
anyhow = "1"
🎯 Summary: Your Rust GUI Chat Client Toolkit
Category | Tool/Libraries | Role |
---|---|---|
Core Language | rustup , cargo | Project management & building |
GUI Framework | egui , eframe | Chat interface and rendering |
Async Runtime | tokio | TCP networking and background tasks |
Data Channels | tokio::sync::mpsc | Send/receive between GUI and network |
Helpers | anyhow , serde , etc. | Error handling and data formatting |
With these tools in place, you’re fully equipped to build a modern, asynchronous, cross-platform Rust GUI chat client—from backend networking to frontend interface.
❓ How to Set Up the Rust GUI Chat Client Project Structure?
To build a professional and scalable Rust GUI chat client, you need a well-structured project layout that separates your GUI logic, networking code, and message handling.
This clean architecture not only helps with readability and maintenance, but also prepares your codebase for future expansions—such as adding emojis, user lists, WebSocket support, or even a WebAssembly port.
But before we dive into the actual structure, let’s make sure your Rust development environment is properly set up.
📚 Prerequisite: Set Up Your Rust Development Environment First
If you’re using Windows or you’re brand new to Rust, you must ensure your toolchain is ready.
Check out this step-by-step setup guide to avoid common beginner errors:
🔗 Rust Development Environment on Windows – Full Setup Guide
It covers how to install Rust, configure
cargo
, and set up VS Code, so you’re ready to build GUI apps like this chat client without surprises.
🧱 Recommended Project Structure for a Rust GUI Chat Client
Organizing your files properly from the beginning makes development smoother and scaling easier. Here’s a basic structure we recommend:
rust-gui-chat-client/
├── Cargo.toml
├── src/
│ ├── main.rs # Entry point – launches the GUI
│ ├── app.rs # GUI state & layout (egui logic)
│ ├── network.rs # Async TCP networking
│ ├── message.rs # Message structure & formatting
│ └── utils.rs # Optional: timestamps, color formatting, etc.
File | Purpose |
---|---|
main.rs | Starts the GUI app using eframe::run_native() |
app.rs | Stores chat history, input field, render logic |
network.rs | Handles async TCP connections using tokio |
message.rs | Defines your chat message format |
utils.rs | Helper functions (e.g., format_timestamp() ) |
🧑💻 Step-by-Step Setup Guide
✅ 1. Create the Project
cargo new rust-gui-chat-client
cd rust-gui-chat-client
✅ 2. Add Dependencies in Cargo.toml
[package]
name = "rust-gui-chat-client"
version = "0.1.0"
edition = "2021"
[dependencies]
tokio = { version = “1”, features = [“full”] } eframe = “0.27” egui = “0.27” anyhow = “1”
You now have support for GUI rendering, async TCP, and error handling.
✅ 3. Declare Your Modules in main.rs
mod app;
mod network;
mod message;
mod utils;
use app::ChatApp;
fn main() -> eframe::Result<()> {
let options = eframe::NativeOptions::default();
eframe::run_native("Rust GUI Chat Client", options, Box::new(|_cc| Box::new(ChatApp::new())))
}
This launches your app and ties everything together. The
ChatApp
struct will handle UI state and rendering inapp.rs
.
🔍 Why Modular Design Is Important
- Separates responsibilities: GUI code and networking don’t interfere with each other
- Improves testability: You can mock the network or unit-test message logic independently
- Scales better: You can later add features like settings or authentication without breaking everything
- Matches real-world Rust app architecture: Similar to popular Rust GUI and game engines like
bevy
,iced
, anddioxus
🧰 Optional Upgrade: Folder-Based Structure for Larger Projects
If your app starts growing in complexity, you can reorganize it like this:
src/
├── gui/
│ └── mod.rs # Chat UI (egui logic)
├── net/
│ └── mod.rs # TCP networking
├── models/
│ └── message.rs # Shared data structures
├── utils.rs
└── main.rs
This layout mirrors production Rust projects, improves navigation, and supports future team collaboration.
🧠 Developer Tip
Think modular from the start.
Even small apps benefit from clean separation, and it saves time later when debugging or extending features.
✅ Summary
To recap, here’s what you should do to set up your Rust GUI chat client project correctly:
- Follow this setup guide to prepare your Rust environment.
- Create a new project and add the right dependencies.
- Break your code into logical modules:
app
,network
,message
, andutils
. - Prepare for future growth with a clean file structure.
❓ How to Implement the Async TCP Connection in Your Rust GUI Chat Client?
To implement a real-time chat experience in your Rust GUI chat client, you need to establish an asynchronous TCP connection that runs independently from the GUI.
This allows users to send and receive messages without freezing or blocking the user interface—thanks to tokio
, Rust’s powerful async runtime.
In this section, you’ll build the networking layer that connects your client to a server using TcpStream
, receives incoming messages, and forwards outgoing ones—all while keeping the UI reactive.
🧠 Why Async TCP Is Essential in a GUI Chat App
Rust GUIs built with egui
run on a render loop, updating the screen frame-by-frame. If you block the main thread waiting for a message, the UI will freeze.
Using async TCP with tokio
allows your chat client to:
- 📥 Receive messages in the background
- 📤 Send messages when the user presses “Send”
- 🌀 Update the GUI without lag
That’s why we’ll use tokio::spawn()
to offload all network operations onto background tasks.
🔌 Step-by-Step: Creating the TCP Networking Module
✅ 1. Create src/network.rs
use tokio::{
io::{AsyncBufReadExt, AsyncWriteExt, BufReader},
net::TcpStream,
sync::mpsc::{UnboundedReceiver, UnboundedSender},
};
use std::sync::{Arc, Mutex};
pub async fn start_connection(
address: &str,
tx_gui: Arc<Mutex<Vec<String>>>, // Shared GUI message list
mut rx_gui: UnboundedReceiver<String>, // From GUI to network
) {
match TcpStream::connect(address).await {
Ok(stream) => {
let (read_half, mut write_half) = stream.into_split();
let mut reader = BufReader::new(read_half).lines();
// Spawn a task to handle incoming messages
let incoming_tx = Arc::clone(&tx_gui);
tokio::spawn(async move {
while let Ok(Some(line)) = reader.next_line().await {
let mut messages = incoming_tx.lock().unwrap();
messages.push(line);
}
});
// Main loop: forward GUI messages to the server
while let Some(msg) = rx_gui.recv().await {
let _ = write_half.write_all(format!("{}\n", msg).as_bytes()).await;
}
}
Err(e) => {
let mut messages = tx_gui.lock().unwrap();
messages.push(format!("❌ Failed to connect: {}", e));
}
}
}
🔁 2. Add Channels to Communicate with the GUI
In app.rs
, define:
use tokio::sync::mpsc::{unbounded_channel, UnboundedSender};
use std::sync::{Arc, Mutex};
pub struct ChatApp {
pub messages: Arc<Mutex<Vec<String>>>,
pub input: String,
pub sender: Option<UnboundedSender<String>>,
}
impl ChatApp {
pub fn new() -> Self {
let messages = Arc::new(Mutex::new(vec![]));
let (tx_gui, rx_gui) = unbounded_channel();
let net_messages = Arc::clone(&messages);
tokio::spawn(async move {
crate::network::start_connection("127.0.0.1:8080", net_messages, rx_gui).await;
});
ChatApp {
messages,
input: String::new(),
sender: Some(tx_gui),
}
}
}
This structure does the following:
Component | Role |
---|---|
tx_gui (GUI → network) | Sends messages typed by user |
rx_gui (used by network) | Receives those messages and writes to the TCP stream |
Arc<Mutex<Vec<String>>> | Shared message list updated by the network and read by the GUI |
🧑💻 3. Sending a Message From the GUI
Inside the update()
method of your ChatApp
, wire the send button like this:
if ui.button("Send").clicked() {
if let Some(sender) = &self.sender {
let _ = sender.send(self.input.clone());
}
self.input.clear();
}
This forwards the user’s input to the network task, which sends it over TCP. Meanwhile, new messages from the server are pushed into the messages
list, which is read on every frame and displayed in the UI.
✅ Summary: What You’ve Achieved
Component | Result |
---|---|
tokio::spawn() | Runs async networking in the background |
TcpStream::connect() | Establishes TCP connection to server |
BufReader::lines() | Reads messages from the socket |
write_all() | Sends typed messages to server |
mpsc::unbounded_channel() | Bridges GUI and network tasks |
Arc<Mutex<Vec<String>>> | Safely shares chat history between threads |
You now have a fully functioning asynchronous network layer in your Rust GUI chat client.
❓ How to Design a User-Friendly Interface for Your Rust GUI Chat Client Using eGUI?
Designing a user-friendly interface in your Rust GUI chat client involves combining clarity, responsiveness, and simplicity—all of which are made easy with eGUI.
Since eGUI uses an immediate-mode rendering model, the UI is redrawn every frame based on the current state, allowing for fluid updates and real-time feedback without complex event handling.
Whether you’re displaying a list of messages, typing a new one, or clicking “Send”, eGUI enables you to handle it all with minimal code—and maximum flexibility.
🧱 Key Components of a Chat Interface (and How to Build Them in eGUI)
Let’s break down a modern chat UI into parts and implement them one by one using eGUI’s widgets.
💬 1. Scrollable Message Display Panel
Purpose: Show a real-time stream of messages with automatic layout.
egui::ScrollArea::vertical().show(ui, |ui| {
let messages = self.messages.lock().unwrap();
for msg in messages.iter() {
ui.label(msg);
}
});
Feature | Description |
---|---|
ScrollArea::vertical() | Enables scrolling through messages |
label() | Displays each chat message |
self.messages | Shared state updated by the networking thread |
💡 You can style the text later using RichText
to add colors, bold text, or emojis.
✍️ 2. Message Input Field
Purpose: Allow the user to type a message with keyboard focus and Enter key support.
let input = ui.text_edit_singleline(&mut self.input);
if input.lost_focus() && ui.input(|i| i.key_pressed(egui::Key::Enter)) {
self.send_message();
}
💡 This gives a natural typing experience and lets the user press Enter to send without needing to click.
📤 3. Send Button (Optional UI Redundancy)
Purpose: Offer an accessible button for mouse users.
if ui.button("Send").clicked() {
self.send_message();
}
Combine both actions into one function:
impl ChatApp {
fn send_message(&mut self) {
if !self.input.trim().is_empty() {
if let Some(sender) = &self.sender {
let _ = sender.send(self.input.clone());
}
self.input.clear();
}
}
}
🧭 4. Layout Tips for Better UX
Layout Element | UX Benefit |
---|---|
CentralPanel | Keeps content focused in center of the window |
TopBottomPanel | Can be used for title bars or connection status |
Spacing::default() | Prevents components from feeling cramped |
ctx.request_repaint() | Ensures continuous refresh (already automatic in eframe ) |
egui::CentralPanel::default().show(ctx, |ui| {
ui.heading("📨 Rust Chat Client");
ui.separator();
// Scrollable messages
// Input box and send button here
});
🎨 5. Optional UI Enhancements with RichText
use egui::RichText;
ui.label(RichText::new("Server: Connected").strong().color(egui::Color32::GREEN));
Add visual feedback for:
- Connection status
- Error messages
- Usernames (bold or colored)
- Time formatting (
HH:MM
)
📱 6. Responsive Layout Suggestions
- Use
egui::ScrollArea
for large message lists - Set
min_height
ormax_height
for input box - Avoid absolute positioning—use flexible UI layout patterns
- Consider font scaling for accessibility (e.g., larger fonts on high DPI)
✅ Summary: Your eGUI Chat Interface Blueprint
UI Element | Widget(s) Used |
---|---|
Chat history panel | ScrollArea , label() |
Message input | text_edit_singleline() |
Send button | button() + clicked() |
Layout container | CentralPanel , TopBottomPanel |
With just a few lines of code, you now have a fully interactive, real-time chat interface in Rust.
❓ How to Send and Receive Messages in a Rust GUI Chat Client?
Sending and receiving messages in your Rust GUI chat client involves connecting the user input with the async TCP stream and updating the GUI in real time as new messages arrive.
Thanks to Rust’s async architecture and eGUI’s immediate-mode UI, you can process user actions and server responses smoothly—without freezing the interface or losing performance.
Let’s connect all the moving parts: the message input box, the send button, the networking task, and the shared message list.
🧭 Message Flow Overview
Before we code, here’s the high-level flow:
User Input (GUI) → Sender Channel → TCP Socket → Server
Incoming TCP Data → Receiver Task → Message List → GUI Display
Two separate channels keep your GUI and network code independent but synchronized.
📤 Sending Messages from GUI to Server
In your ChatApp
struct (defined in app.rs
), we previously created a message sender:
use tokio::sync::mpsc::UnboundedSender;
pub struct ChatApp {
pub input: String,
pub messages: Arc<Mutex<Vec<String>>>,
pub sender: Option<UnboundedSender<String>>,
}
Add a unified send method:
impl ChatApp {
pub fn send_message(&mut self) {
if !self.input.trim().is_empty() {
if let Some(sender) = &self.sender {
let _ = sender.send(self.input.clone());
}
self.input.clear();
}
}
}
Call this method in both cases:
- When pressing Enter
- When clicking the Send button
if input.lost_focus() && ui.input(|i| i.key_pressed(egui::Key::Enter)) {
self.send_message();
}
if ui.button("Send").clicked() {
self.send_message();
}
📥 Receiving Messages from Server to GUI
In your network.rs
file, you should already have this logic inside start_connection()
:
let incoming_tx = Arc::clone(&tx_gui);
tokio::spawn(async move {
while let Ok(Some(line)) = reader.next_line().await {
let mut messages = incoming_tx.lock().unwrap();
messages.push(line);
}
});
This background task listens for messages and appends them to the shared Vec<String>
, which is then rendered by your GUI.
To render them, use this in app.rs
:
egui::ScrollArea::vertical().show(ui, |ui| {
let messages = self.messages.lock().unwrap();
for msg in messages.iter() {
ui.label(msg);
}
});
📌 Thread Safety Reminder
Because GUI and network code access the same data (messages
), we protect it using:
use std::sync::{Arc, Mutex};
Arc
: allows shared ownership across threadsMutex
: ensures only one thread modifies the data at a time
This is a common and safe pattern in Rust GUI apps.
✨ UX Enhancements (Optional)
Feature | Benefit |
---|---|
✅ Auto-scroll to bottom | Keeps latest messages in view |
🕒 Show timestamps | Use chrono to attach time to each message |
🧪 Debug mode | Print logs to console during development |
🟢 Connection status | Display “Connected” or “Disconnected” visually |
Example with timestamp:
let time = chrono::Local::now().format("%H:%M");
let msg = format!("[{}] {}", time, line);
✅ Summary: End-to-End Message Pipeline
Stage | Component |
---|---|
User types message | text_edit_singleline() |
Clicks “Send” | button() → send_message() |
Sent via channel | UnboundedSender<String> |
Written to server | write_all() in network.rs |
Server responds | BufReader::lines() reads input |
Stored in list | Arc<Mutex<Vec<String>>> |
Rendered to UI | ScrollArea::vertical() |
With this pipeline in place, your Rust GUI chat client is now a fully functional real-time messaging app.
❓ How to Keep the Rust GUI Chat Client Interface Responsive and Realtime?
Keeping your Rust GUI chat client responsive and realtime means ensuring that the user interface updates smoothly, even while background tasks like networking are running.
In a chat application, delays or UI freezes quickly frustrate users. Rust’s async features combined with eGUI’s immediate-mode rendering model allow us to avoid such issues.
Let’s explore the best techniques to maintain smooth interactions, avoid blocking, and improve real-time feedback.
🧠 Why Responsiveness Matters
In GUI apps—especially chat clients—users expect:
- ✍️ Instant typing feedback
- 🟢 Real-time message delivery
- ⌛ No frozen windows or delayed responses
- 📜 Auto-scrolling to latest messages
To meet these expectations, your app needs to:
- Run networking logic asynchronously
- Separate UI rendering from message handling
- Ensure non-blocking updates
⚙️ 1. Use tokio::spawn
for Background Networking
If you haven’t already, all your TCP operations should live in a separate async task like this:
tokio::spawn(async move {
start_connection("127.0.0.1:8080", messages, rx_gui).await;
});
This keeps the GUI loop focused entirely on rendering and input.
🔁 2. Call ctx.request_repaint()
to Continuously Refresh UI
eGUI’s eframe
automatically refreshes every frame, but if your UI appears to lag or updates only when the user interacts, force a repaint like this:
ctx.request_repaint();
Place it at the end of your update()
method:
fn update(&mut self, ctx: &egui::Context, _frame: &mut eframe::Frame) {
// UI layout here ...
ctx.request_repaint(); // keeps it updating
}
This is crucial when new messages arrive from a background thread, and the screen needs to reflect it without user input.
📜 3. Auto-Scroll to Latest Message (WIP Tip)
eGUI does not yet offer a built-in “scroll to bottom” feature, but you can:
- Track the total message count
- Keep a
scroll_to_end
flag and adjust layout offsets manually - OR simplify UX by showing newest messages at the top (
.reverse()
)
Experimental:
egui::ScrollArea::vertical()
.auto_shrink([false; 2])
.show(ui, |ui| {
let messages = self.messages.lock().unwrap();
for msg in messages.iter().rev() {
ui.label(msg);
}
});
🧪 4. Add Visual Feedback and States
UX Element | Purpose |
---|---|
“Connecting…” banner | Shows progress at startup |
Color-coded status | Green: Connected, Red: Error |
Placeholder message | “Start typing…” in input field |
Message animations | Fade or timestamp on new message (WIP) |
Use RichText
for visual cues:
use egui::RichText;
ui.label(RichText::new("🟢 Connected").color(egui::Color32::GREEN).strong());
🧹 5. Avoid Blocking Calls in update()
Your update()
method in eGUI must not contain:
std::thread::sleep()
.await
ortokio::join!()
calls- Heavy computation (move it to a background thread)
Instead, update GUI state based on shared variables (Arc<Mutex<T>>
), and let tokio
handle everything async outside the UI.
✅ Summary: How to Maintain Smooth UX in Rust GUI Chat Apps
Technique | Result |
---|---|
Use tokio::spawn | Background networking |
Avoid blocking UI thread | No .await in update() |
Use request_repaint() | Ensure consistent UI refresh |
Provide visual feedback | Show connection or error states |
Use Arc<Mutex<T>> properly | Safe data sharing between tasks |
Responsiveness is the heart of user experience—this section ensures your Rust GUI chat client feels smooth, modern, and professional.
❓ How to Test Your Rust GUI Chat Client and Fix Common Issues?
Testing your Rust GUI chat client involves verifying the message flow, checking the connection behavior, and debugging UI responsiveness or crash errors.
Even if everything compiles correctly, real-world usage often reveals problems that don’t show up until runtime—such as unresponsive inputs, broken connections, or unreadable UI elements.
Let’s walk through how to run effective tests, simulate errors, and fix the most common issues developers face when building chat apps in Rust.
🧪 1. Manual Testing: Localhost Simulation
Start with running everything on your local machine:
✅ Step-by-Step Testing Instructions
- Run the server in one terminal
cargo run --bin server
- Open a new terminal and run the GUI chat client
cargo run --bin gui_client
- Open multiple clients
- Try typing messages in each client.
- Confirm all messages appear across all windows.
- Try sending empty input, emojis, or long strings.
- Disconnect the server
- Close the server terminal.
- See how your client reacts: does it freeze, crash, or display “Disconnected”?
🚨 2. Common Issues and How to Fix Them
❌ Client Doesn’t Connect
Symptoms:
- No message appears
- Terminal logs are blank
- UI shows no indication of connection status
Fix:
- Ensure server is running (
127.0.0.1:8080
) - Add error logging in
network.rs
:
Err(e) => {
let mut messages = tx_gui.lock().unwrap();
messages.push(format!("❌ Failed to connect: {}", e));
}
❌ Messages Aren’t Displaying
Symptoms:
- Input sends, but chat area remains blank
- Only local messages are visible
Fix:
- Confirm that
self.messages.lock()
is being read correctly in GUI - Check that messages are actually being sent into the channel (
sender.send(...)
) - Ensure
ctx.request_repaint()
is being called to refresh the interface
❌ UI Freezes or Lags
Symptoms:
- GUI becomes unresponsive
- Messages delayed or lost
Fix:
- Never use
.await
or.sleep()
in theupdate()
method - Offload heavy work to
tokio::spawn
- Keep UI logic lightweight and state-driven
❌ App Crashes on Empty Message or Special Input
Symptoms:
- Typing Enter with no input crashes the app
- Non-UTF8 input causes panic
Fix:
- Add guard clauses in
send_message()
:
if self.input.trim().is_empty() {
return;
}
- Always handle
.unwrap()
calls cautiously—use.ok()
or.map_err()
🧰 3. Enable Logging for Debugging
Add this to main.rs
for basic logging support:
env_logger::init();
log::info!("Starting chat client...");
In Cargo.toml
:
log = "0.4"
env_logger = "0.10"
You can now log connection attempts, errors, and state changes with:
log::info!("Connected to server");
log::error!("Failed to send message");
🧪 4. Write Integration Tests (Optional)
Create a tests/
directory and simulate client-to-server flows:
mkdir tests
touch tests/integration_test.rs
Use tokio::test
to simulate sending a message to a mock TCP server and asserting results.
🧼 5. Add Visual Indicators for Errors
Show status feedback in the UI with RichText
:
ui.label(RichText::new("❌ Not connected").color(egui::Color32::RED));
Or append server error messages to the message list for full transparency:
self.messages.lock().unwrap().push("⚠️ Server unreachable.".to_string());
✅ Summary: Best Practices for Testing Your Rust GUI Chat App
Task | Tool / Technique |
---|---|
Run server and client locally | Manual testing via cargo run |
Track UI status | RichText , connection labels |
Debug logic flow | log , env_logger , println!() |
Avoid UI freeze | Never block update() |
Verify message sync | Test multiple windows |
Handle disconnects gracefully | Show error and reconnect option (future step) |
Thorough testing turns your chat client from a prototype into a polished user-ready application.
❓ How to Customize and Extend Your Rust GUI Chat Client for Future Features?
Once your Rust GUI chat client is working, the next step is to customize and expand it with real-world features like nicknames, timestamps, themes, or even file sharing.
These improvements not only enhance user experience but also showcase your skills as a Rust developer who understands UI/UX, networking, and scalable architecture.
Let’s explore practical ideas you can build upon the current foundation—with code strategies for each.
🆔 1. Add a Nickname System
By default, the client displays the socket address (127.0.0.1:12345
) as the identifier. You can improve UX by letting users enter a nickname at startup.
✅ UI Input for Nickname
In ChatApp::new()
, add a new field:
pub struct ChatApp {
pub nickname: String,
pub input: String,
pub messages: Arc<Mutex<Vec<String>>>,
pub sender: Option<UnboundedSender<String>>,
}
Prompt for nickname on first run:
if self.nickname.is_empty() {
ui.label("Enter your nickname:");
ui.text_edit_singleline(&mut self.nickname);
if ui.button("Start Chat").clicked() {
// Move to chat interface
}
return;
}
Attach nickname to outgoing message:
let msg = format!("{}: {}", self.nickname, self.input.trim());
🕒 2. Add Timestamps to Messages
Use the chrono
crate:
chrono = "0.4"
When appending a message:
let time = chrono::Local::now().format("%H:%M:%S");
let full_msg = format!("[{}] {}", time, msg);
Result:
[12:45:01] alice: Hello world!
🎨 3. Implement Theme Switching (Light/Dark)
Use egui::Visuals
:
ui.horizontal(|ui| {
if ui.button("Light Mode").clicked() {
ctx.set_visuals(egui::Visuals::light());
}
if ui.button("Dark Mode").clicked() {
ctx.set_visuals(egui::Visuals::dark());
}
});
You can store theme preference in a config file using serde
and ron
or toml
.
📁 4. File Transfer (Advanced)
Rust’s tokio
supports sending binary streams over TCP. You can:
- Add drag-and-drop support to the GUI
- Encode files as bytes and stream them
- Use a secondary TCP port or WebSocket for binary transmission
Not beginner-level, but impressive for a portfolio.
📲 5. Add WebAssembly (WASM) Build Target
Since egui
supports WASM, your app can run in browsers!
✅ Add wasm32
target:
rustup target add wasm32-unknown-unknown
✅ Compile to WASM:
cargo build --target wasm32-unknown-unknown
Then wrap it in eframe::wasm_app
or host it using trunk
.
📦 Potential use case:
Users access your chat app from browsers—no install required.
🌍 6. Add Multi-Room or Group Chat Support
Structure messages as JSON:
{
"room": "general",
"user": "alice",
"message": "Hello!"
}
Parse using serde_json
:
use serde::{Deserialize, Serialize};
#[derive(Serialize, Deserialize)]
struct ChatMessage {
room: String,
user: String,
message: String,
}
This allows room switching and private messaging logic.
✅ Summary: Feature Ideas to Grow Your Rust Chat App
Feature | Benefit |
---|---|
Nicknames | Friendly identity |
Timestamps | Message clarity |
Theme switching | Better UX and accessibility |
File transfer | Real-world functionality |
WASM build | Cross-platform/browser compatibility |
Chat rooms | Group and private conversations |
Extending your Rust GUI chat client into a full-featured messenger app turns it into a serious portfolio project—and prepares you for building production-ready systems.
❓ What Are the Next Steps After Building a Rust GUI Chat Client?
After completing your Rust GUI chat client, it’s time to take your skills to the next level by expanding your project, refining your codebase, and challenging yourself with new Rust-based simulations and real-time systems.
This chat client is more than just a learning project—it’s a functional, real-world application that proves your grasp of Rust’s async runtime, GUI rendering, and networking capabilities.
🔧 1. Refactor, Polish, and Document
Now that your app works:
- 🧹 Clean up the structure: Separate logic cleanly into
network
,gui
,utils
. - 📚 Add documentation: Comment your modules and write a clear
README.md
. - 🧪 Write tests: Especially around message processing or formatting.
- 🚀 Add a license + contribution guide: If sharing on GitHub.
🌐 2. Publish and Showcase
Make your project public:
- Push it to GitHub with a clear structure.
- Include a demo GIF and usage instructions.
- Tag it with
#rust
,#tokio
,#egui
,#chat-client
.
If you want to take it even further, consider turning your client into a WebAssembly app using trunk
and egui
‘s WASM support.
You can host it for free on GitHub Pages or Netlify.
🧠 3. Expand Your Rust Capabilities
This project gives you the confidence to tackle more advanced ideas.
Here are two fun and challenging Rust projects that build on the skills you’ve learned:
☕ Try a State-Based System:
🟢 Rust Coffee Vending Machine Simulator
This project teaches you how to manage application states (e.g., inserting coins, selecting coffee, dispensing change) with Rust’s powerful enums and pattern matching.
Perfect for improving your logic flow and stateful UI management!
🗺️ Dive Into Procedural Systems:
🟢 Rust MUD Game Map System
Learn how to build a text-based game world using coordinates, tile-based mapping, and character interactions.
If you’re interested in building a game or simulation, this is a natural next step from a chat client!
📈 4. Challenge Yourself With Advanced Features
Feature Idea | Tech Needed |
---|---|
Group chat rooms | JSON message parsing (serde ) |
Message encryption | ring , rustls , sodiumoxide |
Real-time notifications | tokio::select! + WebSockets |
Chat history storage | sled , sqlite , or serde_json |
GUI animations | egui::RichText , conditional UI |
✅ Summary
Your journey doesn’t end here—it branches into new Rust challenges, real-time systems, game simulations, and complex GUI apps.
Step | Description |
---|---|
📂 Refactor | Clean up and structure your app |
🌍 Share | Publish to GitHub, write about it |
📦 Build more | Try vending machines, MUDs, or games |
🔁 Iterate | Improve UX, performance, and visuals |
Ready to go deeper into Rust?
👉 Check out Rust Coffee Vending Machine Simulator and Rust MUD Game Map System for your next hands-on challenge.
full code
// Rust GUI Chat Client – Final Algorithmic Breakdown with Full Annotated Code
// Dependencies required in Cargo.toml
/*
[dependencies]
tokio = { version = "1", features = ["full"] }
eframe = "0.27"
egui = "0.27"
anyhow = "1"
*/
use eframe::egui;
use tokio::net::TcpStream;
use tokio::io::{AsyncBufReadExt, AsyncWriteExt, BufReader};
use tokio::sync::mpsc::{unbounded_channel, UnboundedSender, UnboundedReceiver};
use std::sync::{Arc, Mutex};
use std::thread;
// =============== Algorithmic Structure ===============
// 1. Initialize GUI with a shared state (messages, input, sender)
// 2. Set up a channel (mpsc) between GUI and network thread
// 3. Spawn a background task to manage TCP connection (connect, send, receive)
// 4. GUI thread reads messages and updates UI in real time
// 5. User types -> send through channel -> transmitted to server
// ChatApp Struct: Holds shared state and sender
struct ChatApp {
messages: Arc<Mutex<Vec<String>>>, // Shared chat log
input: String, // Current text input
sender: Option<UnboundedSender<String>>, // Sender to networking task
}
impl Default for ChatApp {
fn default() -> Self {
let messages = Arc::new(Mutex::new(Vec::new()));
let (tx, rx) = unbounded_channel::<String>();
let msg_clone = Arc::clone(&messages);
// Start networking in a new thread using Tokio runtime
thread::spawn(move || {
tokio::runtime::Runtime::new().unwrap().block_on(async move {
if let Ok(stream) = TcpStream::connect("127.0.0.1:8080").await {
let (reader, mut writer) = stream.into_split();
let mut lines = BufReader::new(reader).lines();
// Spawn a task for receiving messages
let msg_rx = Arc::clone(&msg_clone);
tokio::spawn(async move {
while let Ok(Some(line)) = lines.next_line().await {
let mut msgs = msg_rx.lock().unwrap();
msgs.push(line);
}
});
// Main loop: send messages from GUI to server
handle_outgoing(writer, rx).await;
} else {
let mut msgs = msg_clone.lock().unwrap();
msgs.push("❌ Failed to connect to server".to_string());
}
});
});
ChatApp {
messages,
input: String::new(),
sender: Some(tx),
}
}
}
// Function to write messages to server
async fn handle_outgoing(mut writer: tokio::net::tcp::OwnedWriteHalf, mut rx: UnboundedReceiver<String>) {
while let Some(msg) = rx.recv().await {
let _ = writer.write_all(format!("{}\n", msg).as_bytes()).await;
}
}
// GUI Logic
impl eframe::App for ChatApp {
fn update(&mut self, ctx: &egui::Context, _frame: &mut eframe::Frame) {
egui::CentralPanel::default().show(ctx, |ui| {
ui.heading("🟢 Rust Chat Client");
// Scrollable chat message area
egui::ScrollArea::vertical().show(ui, |ui| {
let msgs = self.messages.lock().unwrap();
for msg in msgs.iter() {
ui.label(msg);
}
});
ui.separator();
// Text input and send button
ui.horizontal(|ui| {
let input_field = ui.text_edit_singleline(&mut self.input);
// Send on Enter
if input_field.lost_focus() && ui.input(|i| i.key_pressed(egui::Key::Enter)) {
self.send_message();
}
// Send on Button Click
if ui.button("Send").clicked() {
self.send_message();
}
});
});
// Refresh UI every frame
ctx.request_repaint();
}
}
// Logic to send message to networking thread
impl ChatApp {
fn send_message(&mut self) {
if self.input.trim().is_empty() {
return;
}
if let Some(sender) = &self.sender {
let _ = sender.send(self.input.clone());
}
self.input.clear();
}
}
// Entry point for native desktop app
fn main() -> eframe::Result<()> {
let options = eframe::NativeOptions::default();
eframe::run_native(
"Rust GUI Chat Client",
options,
Box::new(|_cc| Box::<ChatApp>::default()),
)
}
🧠 Rust GUI Chat Client – FAQ
1. What is a Rust GUI chat client?
A Rust GUI chat client is a desktop application built in Rust with a graphical interface (like egui
) that allows users to send and receive real-time messages via network sockets.
2. Why use Rust instead of Python or JavaScript for a chat app?
Rust offers superior performance, memory safety, and zero-cost abstractions—ideal for building responsive, secure, and cross-platform chat clients.
3. What libraries are used in this project?
This project uses tokio
for async networking, eframe
/egui
for the GUI, and anyhow
for error handling.
4. Is this chat client cross-platform?
Yes! Rust compiles to native binaries on Windows, macOS, and Linux. With egui
, your GUI works the same across all platforms.
5. Can I run this chat client in a web browser?
Yes. By compiling the app to WebAssembly (wasm32-unknown-unknown
) and using trunk
, you can run it in a browser.
6. Do I need a Rust server to use this client?
Not necessarily. This client can connect to any TCP server that follows a simple text-line protocol, but writing a Rust-based server is ideal for compatibility.
7. How does the chat client stay responsive while networking?
The client uses tokio::spawn()
to run networking tasks asynchronously in the background, ensuring the GUI stays smooth.
8. Can I add features like nicknames or timestamps?
Yes. You can extend the message format using structs and display enhancements using egui::RichText
.
9. How are messages shared between GUI and networking code?
Messages are passed using tokio::sync::mpsc::unbounded_channel()
, and the shared chat log is managed using Arc<Mutex<Vec<String>>>
.
10. Is this app safe for concurrent use?
Yes. Rust’s ownership system and Mutex
ensure thread-safe access to shared resources.
11. How do I handle connection errors or dropped servers?
You can detect failed connections in network.rs
and push a message like ❌ Disconnected
into the GUI’s message list for feedback.
12. Can this app be extended to support file transfer or group chat?
Absolutely. You can use JSON messages for multi-room support or stream file data using additional TCP logic.
13. How do I style the GUI or change themes?
Use ctx.set_visuals(egui::Visuals::dark())
or .light()
to change themes. You can also use RichText
for custom colors and bold text.
14. Is this a good portfolio project for a Rust developer?
Yes! It demonstrates knowledge of async programming, GUI design, thread safety, and architectural separation—great for showcasing on GitHub or LinkedIn.
🔗 Recommended Official Resources
1. Tokio – The Asynchronous Runtime for Rust
Tokio is the official async runtime used in this chat client for non-blocking TCP networking and concurrent task execution.
It powers the real-time message sending and receiving logic with minimal overhead and maximum safety.
✅ Use it to deepen your understanding of:
tokio::spawn()
– for background tasksTcpStream
– for async connectionsmpsc::unbounded_channel()
– for messaging between GUI and network
📚 Official site: https://tokio.rs
2. eGUI & eframe – Immediate Mode GUI for Rust
eGUI is a simple, fast, and fully-Rust GUI framework, while
eframe
wraps it for building native desktop apps.
It’s what powers your chat interface—everything from text input to message scrolling.
✅ Use it to learn about:
egui::CentralPanel
,ScrollArea
for layoutRichText
for styling messages- WASM support and advanced rendering features
📚 Official repository: https://github.com/emilk/egui