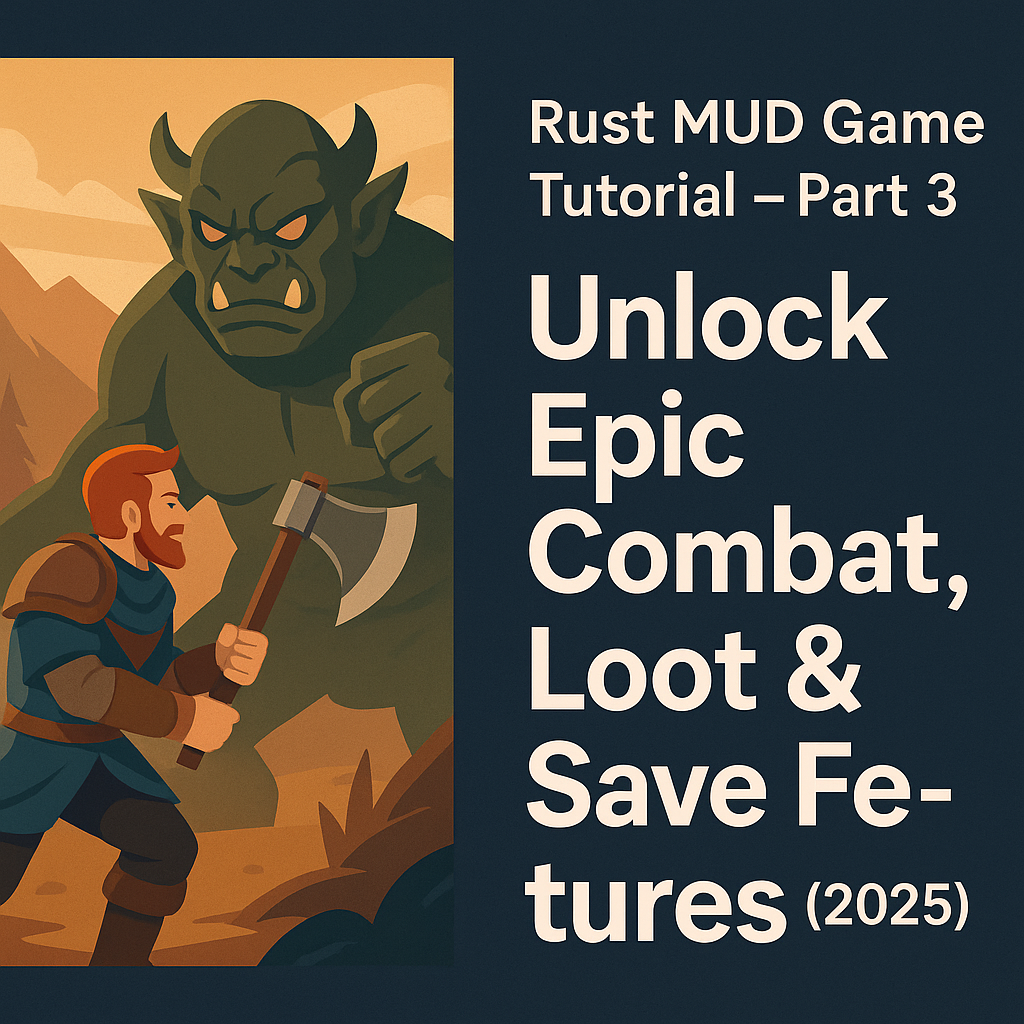
π§© Section 1: Introduction
Rust MUD Game Combat System 2025 begins where Part 2 left off: youβve created rooms, enabled player movement, and built the foundation of a text-based world. Now itβs time to bring danger, tension, and interaction to that worldβby adding monsters, map logic, and a working turn-based battle system.
In this third part of our Rust MUD game tutorial series, weβll design a dynamic map system where each room can contain enemies. Weβll implement a basic monster structure and write reusable combat logic that pits your player against hostile creatures. All of this will be coded in idiomatic, testable, and expandable Rust.
By the end of this guide, youβll be able to:
- Define and connect rooms on a logical game map
- Populate rooms with monsters
- Create a turn-based combat loop with real consequences
- Expand your code into a full RPG framework over time
If you havenβt seen Part 2 yet, read it here first:
π Rust MUD Game Tutorial β Part 2: Player Movement and Room Navigation
Letβs build the core gameplay mechanic that makes MUDs so compelling: the thrill of exploring unknown rooms, encountering creatures, and surviving a strategic battleβall inside your terminal.
Table of Contents
π§ Section 2 : Designing a Dynamic Map System in Rust
A good MUD world isnβt hardcoded. Itβs dynamic, scalable, and logic-driven.
In this section, we implement the core map system, explain every line with detailed comments, and visualize how rooms link and flow.
π§± 1. Room
and GameMap
Structs β With Detailed Comments
use std::collections::HashMap;
/// Represents a single room in the MUD world.
#[derive(Debug)]
struct Room {
/// A short description shown to the player.
description: String,
/// Exits from this room: key = direction ("north", "south"), value = ID of destination room.
exits: HashMap<String, usize>,
}
/// Holds all rooms and manages the map structure.
struct GameMap {
/// Maps unique room ID to the actual Room struct.
rooms: HashMap<usize, Room>,
}
π§ͺ 2. Function to Create a Simple 3-Room World
/// Initializes the game map with three interconnected rooms.
///
/// Layout:
/// [0] Forest Clearing β north β [1] Mountain Path β east β [2] Cave Entrance
fn create_game_map() -> GameMap {
let mut rooms = HashMap::new();
// Room 0: Starting room (forest clearing)
rooms.insert(0, Room {
description: "You are in a quiet forest clearing.".to_string(),
exits: HashMap::from([
("north".to_string(), 1)
]),
});
// Room 1: Path on the mountain
rooms.insert(1, Room {
description: "A narrow path winds up the mountain.".to_string(),
exits: HashMap::from([
("south".to_string(), 0),
("east".to_string(), 2)
]),
});
// Room 2: Entrance to a mysterious cave
rooms.insert(2, Room {
description: "You stand before a dark cave mouth.".to_string(),
exits: HashMap::from([
("west".to_string(), 1)
]),
});
// Return the constructed map
GameMap { rooms }
}
π 3. Algorithm Flow β Room Navigation Logic
START β create_game_map()
ββββββββββββββββββββββββββββββββββββββββββββββββββ
β Room ID 0: "You are in a quiet forest clearing." β
β Exits: north β Room 1 β
ββββββββββββββββββββββββββββββββββββββββββββββββββ
|
| player moves "north"
v
ββββββββββββββββββββββββββββββββββββββββββββββββββββ
β Room ID 1: "A narrow path winds up the mountain." β
β Exits: south β Room 0, east β Room 2 β
ββββββββββββββββββββββββββββββββββββββββββββββββββββ
|
| player moves "east"
v
βββββββββββββββββββββββββββββββββββββββββββββββ
β Room ID 2: "You stand before a dark cave." β
β Exits: west β Room 1 β
βββββββββββββββββββββββββββββββββββββββββββββββ
π§ 4. How to Use This in Your Game Loop
When the player enters a room:
- Fetch room by ID from
GameMap.rooms
- Show
room.description
- List available directions (
room.exits.keys()
) - Wait for player input (e.g., “go north”)
- Lookup next room ID:
room.exits.get("north")
- Move player to new room ID
β Summary
This map system is:
- Fully dynamic (add more rooms easily)
- Decoupled from rendering or input logic
- Expandable for monsters, NPCs, items
In the next section, weβll enhance the world by populating these rooms with monsters using a similar modular pattern.
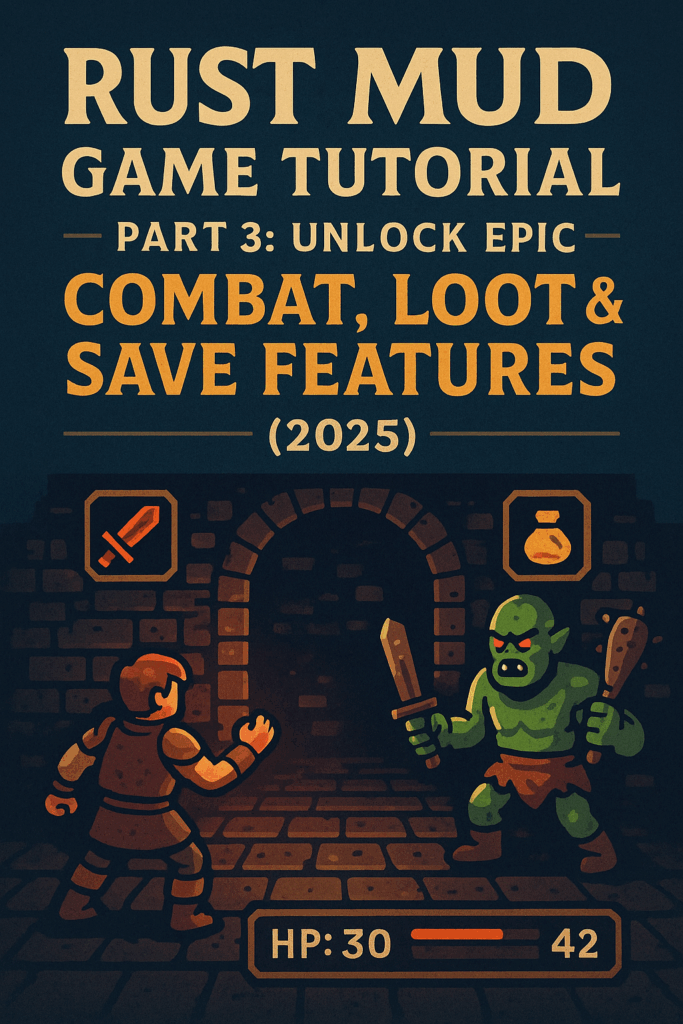
πΎ Section 3: Integrating Monsters into Rooms
A room in a MUD isn’t just a placeβitβs an encounter. Now that we’ve built the basic room system, it’s time to make your world dangerous by populating rooms with monsters. Each monster will have its own stats and will later be used in the combat system.
π§± 1. Define the Monster
Struct
/// A monster that the player can fight.
#[derive(Debug)]
struct Monster {
name: String,
health: i32,
attack: i32,
}
This simple structure stores the monsterβs name, remaining health, and attack power. It will be used during combat to calculate damage and show battle logs.
π§© 2. Update the Room
Struct to Include Monsters
Add a Vec<Monster>
field to the existing Room
struct so that each room can contain one or more enemies.
#[derive(Debug)]
struct Room {
description: String,
exits: HashMap<String, usize>,
monsters: Vec<Monster>, // new field: list of monsters in this room
}
π οΈ 3. Populate Monsters in create_game_map()
Hereβs how to initialize a simple map with monsters:
fn create_game_map() -> GameMap {
let mut rooms = HashMap::new();
// Room 0: Safe zone
rooms.insert(0, Room {
description: "You are in a quiet forest clearing.".to_string(),
exits: HashMap::from([
("north".to_string(), 1)
]),
monsters: vec![],
});
// Room 1: Goblin encounter
rooms.insert(1, Room {
description: "A narrow path winds up the mountain.".to_string(),
exits: HashMap::from([
("south".to_string(), 0),
("east".to_string(), 2)
]),
monsters: vec![
Monster {
name: "Goblin".to_string(),
health: 10,
attack: 3,
}
],
});
// Room 2: Cave monsters
rooms.insert(2, Room {
description: "You stand before a dark cave mouth.".to_string(),
exits: HashMap::from([
("west".to_string(), 1)
]),
monsters: vec![
Monster {
name: "Cave Bat".to_string(),
health: 6,
attack: 2,
},
Monster {
name: "Giant Spider".to_string(),
health: 14,
attack: 4,
}
],
});
GameMap { rooms }
}
π 4. Monster Handling Flow (Logic)
[Player enters room]
β
Check: room.monsters.is_empty()
β
βββββββββββββββββ ββββββββββββββββββββββ
β true β β false β
β (no monsters) β β (initiate combat) β
βββββββββββββββββ ββββββββββββββββββββββ
You now have a map where certain rooms trigger fights automatically based on monster presence.
π‘ Why Use Vec<Monster>
?
- Allows multiple enemies per room
- Enables boss + minion setups
- Makes future combat loops and monster waves possible
β Summary
By the end of this section, your world is no longer empty:
- Rooms contain dangerous enemies
- The game logic can now trigger encounters
- Weβre one step away from a working battle system
π Next up: Section 4: Turn-Based Combat System β where we teach your player to fight back.
βοΈ Section 4: Turn-Based Combat System β Making Fights Real
Youβve built the rooms. Youβve placed the monsters.
Now itβs time to make your game dangerousβfor real.
In this section, weβll implement a reusable, scalable turn-based combat engine where the player and monster exchange blows until one of them falls. This lays the foundation for:
- Boss fights
- Multiple monsters
- Damage calculation logic
- Victory/defeat flow control
π§ 1. Define the Player
Struct
/// Represents the player in the MUD.
#[derive(Debug)]
struct Player {
name: String,
health: i32,
attack: i32,
}
Like Monster
, the Player
has health
and attack
. This symmetry allows a clean combat loop.
π 2. Combat Algorithm Flow (Pseudocode)
function combat(player, monster):
while player.health > 0 AND monster.health > 0:
player attacks monster
if monster dies β break
monster attacks player
if player dies β break
π οΈ 3. Implement the Combat Function
/// Simulates a turn-based fight between player and one monster.
fn combat(player: &mut Player, monster: &mut Monster) {
println!("βοΈ A wild {} appears!", monster.name);
loop {
// --- Player attacks ---
println!("π§ You attack the {}!", monster.name);
monster.health -= player.attack;
println!("π₯ {} takes {} damage! (HP: {})", monster.name, player.attack, monster.health);
if monster.health <= 0 {
println!("β
You defeated the {}!\n", monster.name);
break;
}
// --- Monster attacks ---
println!("πΎ The {} attacks you!", monster.name);
player.health -= monster.attack;
println!("π’ You take {} damage! (HP: {})", monster.attack, player.health);
if player.health <= 0 {
println!("β οΈ You were defeated by the {}...\n", monster.name);
break;
}
println!("βββ Next turn βββ\n");
}
}
π§ͺ 4. Example Usage
fn main() {
let mut player = Player {
name: "Hero".to_string(),
health: 30,
attack: 5,
};
let mut goblin = Monster {
name: "Goblin".to_string(),
health: 12,
attack: 4,
};
combat(&mut player, &mut goblin);
if player.health > 0 {
println!("π You survived the battle!");
} else {
println!("π Game over...");
}
}
π 5. Combat Flowchart (Text)
[Start combat]
β
[Player attacks Monster]
β
[Monster HP <= 0?] ββ Yes β Victory
β No
[Monster attacks Player]
β
[Player HP <= 0?] ββ Yes β Defeat
β No
[Next turn β loop]
π§ Best Practices and Scalability
Feature | Status | Future Possibility |
---|---|---|
Damage calc | βοΈ Basic (flat) | % critical, miss, armor |
Turn order | βοΈ Fixed (player first) | Speed-based initiative |
Multiple enemies | β Not yet | Loop over Vec<Monster> |
Escape options | β Not yet | Add input logic |
You now have a fully working combat loop.
In the next section, weβll show how to trigger this combat when the player enters a room with monsters, and how to handle removing defeated monsters from the room.
π§© Section 5: Connecting Combat to Room Encounters
Combat doesnβt exist in a vacuum.
In an actual MUD, monsters are tied to rooms, and battles are triggered automatically when the player walks into danger.
This section connects everything:
- Map πΊοΈ
- Player π§
- Monsters πΎ
- Combat βοΈ
All into a single loop of exploration and survival.
π§± 1. Define the Game State (Player, Map, Current Room)
struct GameState {
player: Player,
map: GameMap,
current_room: usize,
}
This structure tracks where the player is and gives access to rooms and combat.
π 2. Room Entry Logic with Combat Trigger
/// Handles what happens when a player enters a room.
///
/// If the room contains monsters, trigger combat with each one.
fn handle_room_entry(state: &mut GameState) {
let room = state.map.rooms.get_mut(&state.current_room).unwrap();
println!("\nπ You enter a room:");
println!("{}", room.description);
if room.monsters.is_empty() {
println!("ποΈ The room is quiet.");
return;
}
println!("β οΈ You see {} monster(s):", room.monsters.len());
for m in &room.monsters {
println!("- {}", m.name);
}
// Trigger combat with each monster in order
let mut survivors: Vec<Monster> = vec![];
for mut monster in room.monsters.drain(..) {
combat(&mut state.player, &mut monster);
if monster.health > 0 {
survivors.push(monster); // Monster survived
}
if state.player.health <= 0 {
println!("π You died during the encounter.");
break;
}
}
// Only surviving monsters remain in the room
room.monsters = survivors;
}
π§ͺ 3. Example of Exploration Loop
fn explore(mut state: GameState) {
use std::io::{stdin, stdout, Write};
loop {
handle_room_entry(&mut state);
if state.player.health <= 0 {
println!("π§Ό Respawn system not implemented yet. Game over.");
break;
}
let room = state.map.rooms.get(&state.current_room).unwrap();
println!("\nExits:");
for (dir, id) in &room.exits {
println!("- {} (to Room {})", dir, id);
}
print!("\nWhich direction? ");
stdout().flush().unwrap();
let mut input = String::new();
stdin().read_line(&mut input).unwrap();
let direction = input.trim();
match room.exits.get(direction) {
Some(&next_id) => {
state.current_room = next_id;
}
None => {
println!("β Invalid direction.");
}
}
}
}
π 4. Flow Diagram (Text)
[Player enters room]
β
[Room has monsters?]
β
βββββββββββββββββ
β Yes: Combat β
βββββββββββββββββ
β
[Surviving monsters β back in room]
[Dead monsters β removed]
β
[Display exits β wait for input]
β
[Move to next room β repeat]
β Summary
You now have:
- A dynamic room entry system
- Monster-triggered combat on arrival
- Monsters removed after death
- Loop that allows exploring, fighting, and progressing
In the next section, weβll discuss loot drops, leveling up, and XPβwhat happens after the fight.
π Section 6: Loot Drops and Experience System
A battle means little without rewards.
In this section, we build an item drop system and a basic RPG-style XP + level-up engine, giving purpose and progress to every fight.
π§± 1. Add Item
and Expand Player
/// Lootable item dropped by a monster.
#[derive(Debug, Clone)]
struct Item {
name: String,
value: u32, // currency, score, or future use
}
/// Player now has XP and inventory
#[derive(Debug)]
struct Player {
name: String,
health: i32,
attack: i32,
xp: u32,
level: u32,
inventory: Vec<Item>,
}
πΎ 2. Add loot
and xp_reward
to Monster
#[derive(Debug)]
struct Monster {
name: String,
health: i32,
attack: i32,
loot: Option<Item>,
xp_reward: u32,
}
Now each monster can drop one item and give XP.
π§ͺ 3. Grant Loot and XP After Combat
fn gain_rewards(player: &mut Player, monster: &Monster) {
// Gain XP
player.xp += monster.xp_reward;
println!("π― Gained {} XP!", monster.xp_reward);
// Check for level up (simple threshold system)
let level_threshold = player.level * 20;
if player.xp >= level_threshold {
player.level += 1;
player.health += 10; // heal on level up
println!("π Leveled up to Level {}! Max HP increased!", player.level);
}
// Get loot if any
if let Some(item) = &monster.loot {
player.inventory.push(item.clone());
println!("π You found a {}!", item.name);
}
}
βοΈ 4. Update combat()
to Include Reward Logic
fn combat(player: &mut Player, monster: &mut Monster) {
println!("βοΈ A wild {} appears!", monster.name);
loop {
// Player attacks
println!("π§ You attack the {}!", monster.name);
monster.health -= player.attack;
println!("π₯ {} takes {} damage!", monster.name, player.attack);
if monster.health <= 0 {
println!("β
You defeated the {}!", monster.name);
gain_rewards(player, monster); // β NEW
break;
}
// Monster attacks
println!("πΎ The {} attacks you!", monster.name);
player.health -= monster.attack;
println!("π’ You take {} damage! (HP: {})", monster.attack, player.health);
if player.health <= 0 {
println!("β οΈ You were defeated...");
break;
}
println!("βββ Next turn βββ\n");
}
}
π 5. Flowchart: Combat β Reward β Level Up
[Monster dies]
β
[+ XP β check level up]
β
[+ Loot β add to inventory]
π¦ 6. Example Monster With Loot
Monster {
name: "Goblin".to_string(),
health: 12,
attack: 4,
loot: Some(Item {
name: "Rusty Dagger".to_string(),
value: 10,
}),
xp_reward: 15,
}
β Summary
You now have:
- Monsters that drop loot
- Players who gain XP and level up
- An inventory system that grows with every fight
- A reason to survive beyond just staying alive
In the next section, weβll expand the inventory into an item usage system, letting players heal, equip, or examine loot.
π§ͺ Section 7: Item Use and Equipment Mechanics
Loot is meaningless unless you can use it.
In this section, we turn passive inventory into interactive strategy by building:
- Consumable item usage (e.g., potions)
- Equippable weapons or armor
- A system for applying item effects in battle or between fights
π§± 1. Update Item
Type: Add Categories
#[derive(Debug, Clone)]
enum ItemType {
Consumable,
Weapon,
Armor,
}
#[derive(Debug, Clone)]
struct Item {
name: String,
value: u32,
item_type: ItemType,
effect: i32, // healing or attack bonus
}
Consumable
: heals HP (e.g., potion)Weapon
: increases attackArmor
: (optional) increases defense (future extension)
π§ 2. Update Player
Struct for Equipment
#[derive(Debug)]
struct Player {
name: String,
health: i32,
attack: i32,
xp: u32,
level: u32,
inventory: Vec<Item>,
equipped_weapon: Option<Item>,
}
π 3. Use Consumable Items
fn use_item(player: &mut Player, item_name: &str) {
if let Some(index) = player.inventory.iter().position(|item| item.name == item_name) {
let item = player.inventory.remove(index);
match item.item_type {
ItemType::Consumable => {
player.health += item.effect;
println!("π You used {} and healed {} HP!", item.name, item.effect);
}
ItemType::Weapon => {
player.attack += item.effect;
player.equipped_weapon = Some(item.clone());
println!("π‘οΈ You equipped {} (+{} attack)!", item.name, item.effect);
}
_ => {
println!("β Item effect not implemented.");
}
}
} else {
println!("β Item not found in inventory.");
}
}
π§ͺ 4. Example Items
Item {
name: "Healing Herb".to_string(),
value: 5,
item_type: ItemType::Consumable,
effect: 10, // heal 10 HP
}
Item {
name: "Iron Sword".to_string(),
value: 20,
item_type: ItemType::Weapon,
effect: 4, // +4 attack
}
π 5. Flowchart: Inventory β Use Item β Apply Effect
[Player inventory]
β
[Select item by name]
β
[Item type?]
ββ Consumable β Heal HP
ββ Weapon β Equip and update attack stat
β Summary
With item usage, your game becomes strategic:
- Heal before a tough fight
- Equip stronger weapons to evolve your build
- Make inventory choices matter
In the next section, weβll implement saving and loading game state, so players can return to their progress and continue their adventure.
πΎ Section 8: Saving and Loading Game State in Rust
Your game world isnβt truly alive unless it remembers. In this section, youβll implement a full save/load system that lets players preserve their progress, including player stats, inventory, equipped items, and current location. The implementation will rely on JSON serialization using the serde
ecosystem.
π§± 1. Add Dependencies
To begin, add the following dependencies to your Cargo.toml
:
[dependencies]
serde = { version = "1.0", features = ["derive"] }
serde_json = "1.0"
These enable easy serialization and deserialization of Rust structs.
π§ 2. Make Game Structures Serializable
Update your main game data structures with #[derive(Serialize, Deserialize)]
:
use serde::{Serialize, Deserialize};
#[derive(Debug, Serialize, Deserialize)]
struct Player {
name: String,
health: i32,
attack: i32,
xp: u32,
level: u32,
inventory: Vec<Item>,
equipped_weapon: Option<Item>,
}
#[derive(Debug, Serialize, Deserialize, Clone)]
struct Item {
name: String,
value: u32,
item_type: ItemType,
effect: i32,
}
#[derive(Debug, Serialize, Deserialize, Clone)]
enum ItemType {
Consumable,
Weapon,
Armor,
}
Youβll also need to derive the same traits for Room
, Monster
, GameMap
, and GameState
.
πΎ 3. Saving the Game to a File
Create a function to serialize the GameState
and save it as JSON:
use std::fs::File;
use std::io::Write;
fn save_game(state: &GameState, path: &str) {
let json = serde_json::to_string_pretty(state).unwrap();
let mut file = File::create(path).unwrap();
file.write_all(json.as_bytes()).unwrap();
println!("πΎ Game saved to {}", path);
}
This function saves the game state into a human-readable and easily restorable format.
π 4. Loading a Saved Game
You can now load a game from disk with this function:
use std::fs;
fn load_game(path: &str) -> Option<GameState> {
let data = fs::read_to_string(path).ok()?;
let state: GameState = serde_json::from_str(&data).ok()?;
println!("π Game loaded from {}", path);
Some(state)
}
It safely returns None
if the file or deserialization fails.
π 5. Save/Load System Flow
[Player starts game]
β
[Load Game] β Reads and parses savegame.json
β
[Explore, Fight, Collect]
β
[Save Game] β Serializes GameState to savegame.json
This cycle gives players a sense of persistence and progress.
π§ͺ 6. Sample Integration
You can integrate save/load logic into your main game loop:
fn main() {
let state = if let Some(loaded) = load_game("savegame.json") {
loaded
} else {
GameState::new() // Your default starting state
};
explore(state); // Main gameplay loop
save_game(&state, "savegame.json"); // Save on exit
}
This way, players can resume where they left off.
β Summary
Your Rust MUD now has:
- Full game state persistence
- Player XP, inventory, level, locationβall saved
- Easy integration with future features like checkpoints or autosave
In the next section, weβll polish the user experience with terminal UI enhancements such as colored output, input prompts, and clean formatting.
π¨ Section 9: Terminal UI and Game Polish
At this stage, your Rust MUD game has solid mechanicsβexploration, combat, loot, and saving. But gameplay isnβt just logic. Itβs experience. In this section, we focus on visual and usability improvements that make your terminal game more enjoyable, more readable, and ultimately more engaging.
β¨ 1. Add Color with colored
Crate
Terminal color can emphasize key actions like damage, healing, or level-ups.
Add to Cargo.toml
:
colored = "2.0"
Example Usage:
use colored::*;
println!("{}", "You defeated the goblin!".green().bold());
println!("{}", "You took 10 damage!".red());
println!("{}", "Level Up!".yellow().bold());
This immediately makes important feedback stand out from standard text.
π 2. Improve Readability with Line Breaks and Headers
Structure the output into clearly separated sections:
println!("{}\n{}", "π You enter a room:".bold(), room.description);
println!("\nAvailable exits:");
for (dir, id) in &room.exits {
println!(" β {} (Room {})", dir.cyan(), id);
}
Break up long text with newlines, emojis, or box-style formatting if needed.
β¨οΈ 3. Add Clear Input Prompts
use std::io::{stdin, stdout, Write};
print!("β‘οΈ What will you do next? ");
stdout().flush().unwrap();
Always flush the prompt and avoid stacking input lines without context.
π§ 4. Add Symbols and Icons to Represent Actions
Use emojis or ASCII art to quickly convey meaning:
Symbol | Meaning |
---|---|
βοΈ | Combat begins |
π©Έ | HP or damage |
π | Loot drop |
π§ͺ | Item usage |
πΎ | Game saved |
π | Game loaded |
π§ | Player status |
This helps players mentally scan events and reactions faster.
π 5. Optional: Clear the Screen on New Room Entry
This makes each room feel like a new scene:
print!("\x1B[2J\x1B[1;1H"); // ANSI escape to clear screen
You can also encapsulate this in a helper function like fn clear_screen()
.
π 6. Display Player Stats with Style
Show live stats after each turn or action:
fn display_status(player: &Player) {
println!(
"\nπ§ {} | HP: {} | ATK: {} | LVL: {} | XP: {}",
player.name.bold(),
player.health.to_string().red(),
player.attack.to_string().blue(),
player.level.to_string().yellow(),
player.xp.to_string().cyan()
);
}
This creates a clean heads-up display that can be reused anywhere.
β Summary
With terminal polish, your game transitions from “tech demo” to “playable experience”. Youβve now added:
- Full-color terminal output
- Clear prompts and status sections
- Icons and structured visual feedback
- Readable, modern UX in pure text
In the final section, weβll wrap everything into a launchable game: handling errors, organizing the codebase, and preparing for future features like NPCs or multiplayer.
π Section 10: Final Integration and Future Expansion
Your Rust MUD is now fully functionalβwith a world, monsters, combat, loot, saving, and visual polish. In this final section, weβll cleanly integrate all components, handle edge cases, and outline where you can take the game next.
π§© 1. Structure Your Project for Scalability
Organize your codebase using modules:
src/
βββ main.rs
βββ game_state.rs
βββ combat.rs
βββ map.rs
βββ player.rs
βββ items.rs
βββ ui.rs
Each file should contain relevant structs, impl blocks, and helper functions. Use mod
and pub
to expose interfaces.
Example:
// In combat.rs
pub fn combat(player: &mut Player, monster: &mut Monster) { ... }
π§ͺ 2. Add Error Handling for Robustness
Avoid .unwrap()
in production-level code. Instead, use:
match File::create("save.json") {
Ok(mut file) => { ... }
Err(e) => eprintln!("Error saving game: {}", e),
}
For deserialization:
let result: Result<GameState, _> = serde_json::from_str(&data);
match result {
Ok(state) => { ... }
Err(e) => println!("Failed to load save: {}", e),
}
πΊοΈ 3. Plan for Future Features
Hereβs a roadmap for expanding your MUD beyond this tutorial:
Feature | Description |
---|---|
NPCs & Dialogue | Add interactive characters with branching text |
Quests | Reward-based missions and conditions |
Shops & Economy | Buy/sell items using in-game currency |
Inventory UI | Paginated or filtered inventory views |
Combat Depth | Skills, spells, multi-target attacks |
Multiplayer | Add TCP sockets for real-time interaction |
Map Files | Load maps from JSON/TOML files externally |
π 4. Publish Your Game
- Export your project to GitHub
- Write a
README.md
with features and controls - Add build instructions using
cargo
- Create a binary with
cargo build --release
Consider publishing to:
β Final Summary
Youβve now built a complete terminal-based Rust game with:
- Dynamic world exploration
- Monster encounters and turn-based combat
- Loot and experience systems
- Item usage and equipment
- Save/load functionality
- Visual polish and extensibility
This isnβt just a demoβitβs a foundation for a full RPG or text-based engine.
The future of this MUD is entirely in your hands.
β Frequently Asked Questions (FAQ)
1. What is a MUD game?
A MUD (Multi-User Dungeon) is a text-based online role-playing game where players explore rooms, battle monsters, and interact with the world using typed commands.
2. Why build a MUD game in Rust?
Rust offers memory safety, high performance, and excellent tooling. It’s ideal for building scalable systems like MUD engines without worrying about garbage collection or crashes.
3. Can this MUD game be turned into a multiplayer experience?
Yes. With Rustβs async ecosystem (e.g., tokio
), you can turn this game into a multiplayer server using TCP sockets.
4. How do I save and load the game?
Game state is saved as JSON using the serde_json
crate. Loading reads the file and restores the playerβs progress, inventory, and current location.
5. How does the combat system work?
Combat is turn-based. The player and monster take alternating turns, dealing damage until one sideβs HP reaches zero.
6. Can I use this system to build a full RPG?
Absolutely. The system is modular and scalable. You can add quests, skills, NPCs, or even procedural map generation.
7. Is there support for items like weapons or potions?
Yes. The inventory system supports both consumable and equippable items with effects like healing or increasing attack.
8. What does the map system look like?
Rooms are connected using a HashMap<String, usize>
to represent exits. Each room can have monsters, items, and descriptions.
9. How do I add new rooms or monsters?
Simply insert new entries in the GameMap
‘s room HashMap
and define new Monster
structs with custom stats and loot.
10. Can I use this as a learning project for Rust?
Definitely. It covers many core Rust concepts: ownership, borrowing, structs, enums, pattern matching, file I/O, and more.
11. How do I display colored text in the terminal?
Use the colored
crate. It allows you to style text with colors, bold, and formatting for better readability.
12. Is this MUD engine cross-platform?
Yes. It runs on any system that supports Rust and a terminalβLinux, macOS, and Windows.
13. Can I load maps from external files?
Yes. With minimal changes, you can deserialize room and map data from .json
or .toml
files using serde
.