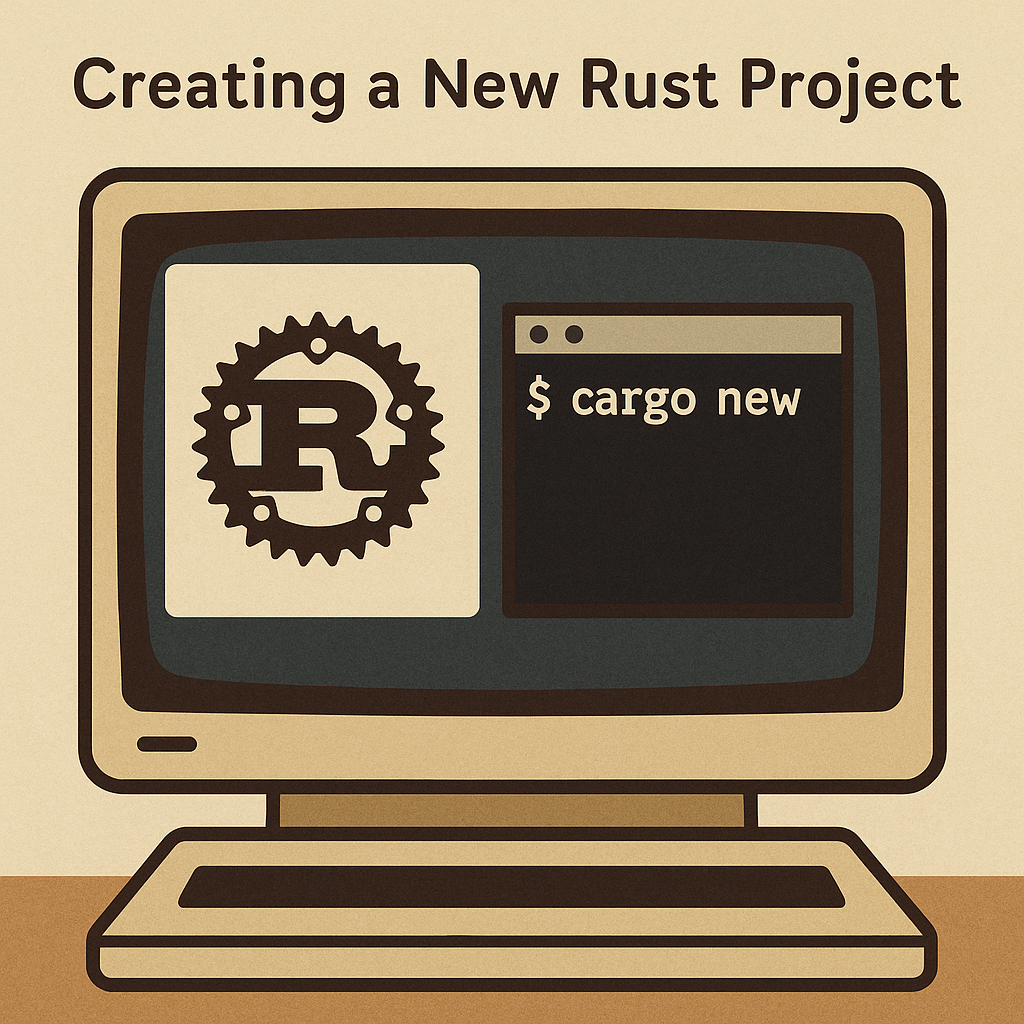
Rust Amazing Grace code tutorial — In the world of creation, ideas are like sparks.
They appear without warning — bright, brilliant, and fleeting.
The difference between those who dream and those who achieve?
It’s not talent.
It’s not luck.
It’s the ability to act immediately.
If you’ve already explored projects like the Rust Coffee Vending Machine Simulator,
the Rust MUD Game Map System,
or the Rust Text-Based 2D Game Tutorial Introduction,
you’ll know this truth well:
the faster you act on your ideas, the faster you grow.
The fastest way to evolve as a creator is to capture ideas the moment they strike.
When you think of something, you must code it right away.
No planning. No waiting. No perfectionism.
Each thought you turn into code — even if it’s rough, even if it’s incomplete —
is a building block in your personal fortress of mastery.
Coding on instinct trains your mind to bridge imagination and execution without fear.
That’s why, in this tutorial, we won’t just “study” how to generate sound in Rust.
We’ll feel a timeless melody — Amazing Grace —
and immediately translate it into code, one note at a time.
Because coding your thoughts at the speed of your ideas — that is the path to true growth.
That is how you transform from a coder into a creator.
Let’s chase inspiration together — and make it real. 🎶🚀
Table of Contents
📚 Step 1: Rust Amazing Grace code tutorial: Setting Up the Project Environment
In this first step of our Rust Amazing Grace code tutorial, we’re not just setting up a project —
we’re preparing a clean, empty canvas where raw thought can be immediately translated into living sound.
Speed is key.
You shouldn’t spend an hour deciding on folder names or wondering which framework to use.
When you have an idea, you move. You act. You code.
So let’s move fast and set up the basics.
🚀 Setting Up the Project
Open your terminal and create a new Rust project:
cargo new rust-amazing-grace
cd rust-amazing-grace
cargo new
creates a new Rust application.cd rust-amazing-grace
navigates into your project folder.
🎶 Adding the Sound Library
We’ll use a lightweight Rust library called rodio
to generate and play pure sound waves — no sound files needed.
In the same terminal, add rodio
:
cargo add rodio
If cargo add
isn’t available yet, install it first:
cargo install cargo-edit
Or, manually add rodio
to your Cargo.toml
file:
[dependencies]
rodio = "0.17" # Or the latest stable version
✅ This step ensures you have everything needed to create real sound with Rust.
📂 Your Basic Project Structure
After setup, your project folder should look like this:
rust-amazing-grace/
├── Cargo.toml
└── src/
└── main.rs
- Cargo.toml manages your dependencies (like rodio).
- src/main.rs is where your Rust code lives — and where your thoughts will become music.
🔥 Why This Fast Setup Matters
When inspiration hits, hesitation kills.
Setting up a new project in under 2 minutes builds a habit:
“I have an idea → I can act on it → I can build it now.”
You don’t just practice coding.
You practice momentum.
And momentum creates mastery.
✅ At this point, your environment is ready.
✅ You’re ready to turn pure thought into pure sound.
Task | Status |
---|---|
New Rust project created | ✅ |
rodio added as a dependency | ✅ |
Project folder ready | ✅ |
Mindset ready for instant action | ✅ |
🚀 Next Step
👉 Step 2: Rust Amazing Grace code tutorial: Understanding How to Create Sound
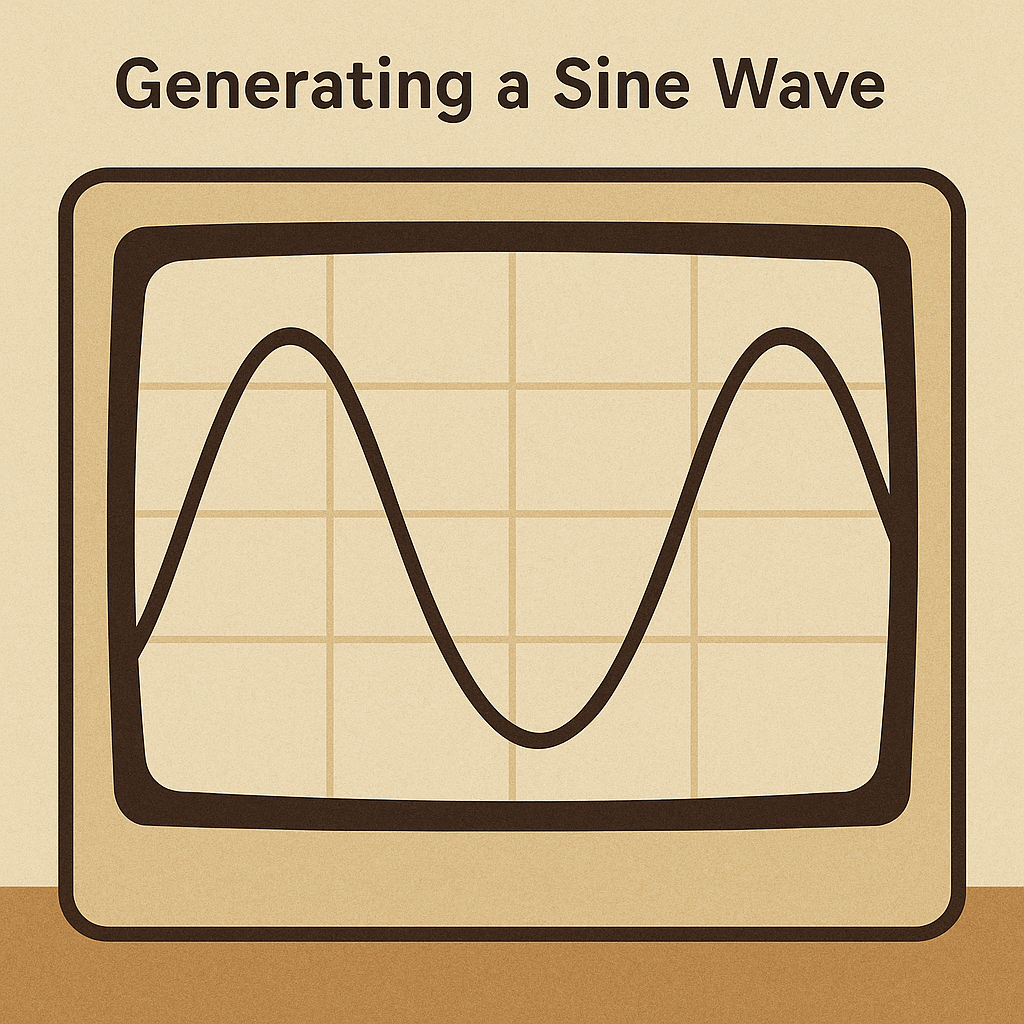
📚 Step 2: Rust Amazing Grace code tutorial: Understanding How to Create Sound
Now that our project environment is ready, it’s time to understand the real magic behind this Rust Amazing Grace code tutorial —
how we can create pure sound directly from code, without using any pre-recorded files.
We aren’t playing existing music.
We are generating music, note by note, from pure math.
🎵 What Is a Sine Wave?
At the heart of all natural sound is the sine wave —
a simple, smooth oscillation that defines the most basic, pure tone a human ear can hear.
In Rust, thanks to the rodio
library, we can create a SineWave source easily:
this simulates the exact physical phenomenon of vibration through air.
When we hear a specific musical note, it’s because air molecules vibrate at a specific frequency (measured in Hertz, Hz).
For example:
- 440 Hz = “A4” (the musical note ‘La’)
- 261 Hz = “C4” (Middle C, ‘도’)
Thus:
A sound = A specific frequency + A duration of vibration
🛠️ How We Create Sound in Rust
In code, we can:
- Generate a pure sine wave at a given frequency (using
SineWave::new(frequency)
). - Play that sound for a set duration (e.g., 500 milliseconds).
- Chain multiple notes together to form a melody.
No audio files.
No fancy music engines.
Just raw frequencies → vibration → music.
✨ Simple Example: Making a Single Sound
Let’s try creating a single note (A4 = 440Hz) for 2 seconds:
use rodio::Source;
use std::{thread, time::Duration};
fn main() {
let device = rodio::default_output_device().expect("No audio output device available!");
let source = rodio::source::SineWave::new(440) // 440 Hz = A4 note
.take_duration(Duration::from_secs(2)) // Play for 2 seconds
.amplify(0.20); // Lower the volume to 20%
rodio::play_raw(&device, source.convert_samples());
thread::sleep(Duration::from_secs(2)); // Wait for the sound to finish
}
✅ This tiny code creates real, physical sound from pure numbers.
🎯 Why This Is Powerful
This simple trick — creating a vibration mathematically —
is the foundation of all digital music production today.
By understanding and controlling frequency and timing,
you can create anything:
- Melodies
- Chords
- Harmonies
- Even your own unique musical experiments
This is true coding creativity at its core.
Concept | Summary |
---|---|
Sound = Frequency + Time | ✅ |
SineWave represents a pure tone | ✅ |
We can code each note directly | ✅ |
No need for audio files | ✅ |
✅ Now you fully understand how we’re going to build Amazing Grace from scratch —
one simple, beautiful note at a time.
🚀 Next Step
👉 Step 3: Rust Amazing Grace code tutorial: Mapping Notes to Frequencies
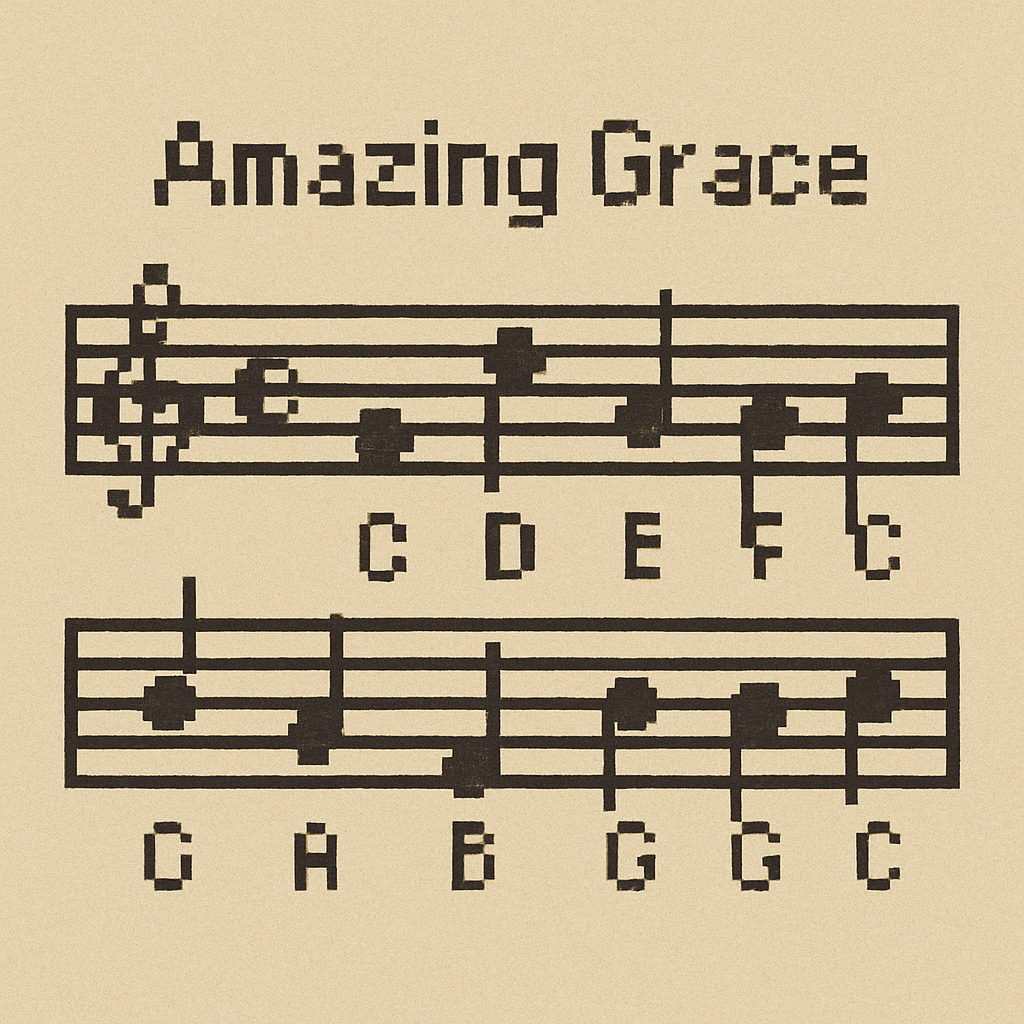
📚 Step 3: Rust Amazing Grace code tutorial: Mapping Notes to Frequencies
In this step of our Rust Amazing Grace code tutorial, we move closer to giving life to the melody inside our minds.
Now that you know how pure sound is generated using sine waves,
it’s time to break down Amazing Grace — one of the most iconic melodies ever written — into pure frequencies that we can code.
The magic lies in understanding:
Music = Numbers.
Numbers = Sound.
Sound = Code.
🎵 Mapping Notes to Frequencies: How Music Becomes Code
Every musical note corresponds to a specific vibration speed, measured in Hertz (Hz).
For example:
- “C4” (Middle C) vibrates at 261 Hz.
- “E4” vibrates at 329 Hz.
- “G4” vibrates at 392 Hz.
Thus, if we know the sequence of notes for a song,
we can map each note to a frequency, and then use SineWave::new(frequency)
to create sound directly from code.
📚 Helpful References for This Step
Here are excellent sources to guide you:
- Amazing Grace Sheet Music (8notes.com) — Review the basic melody and note sequence.
- rodio Official Documentation (docs.rs) — Understand how to use
SineWave
and control playback. - Wikipedia: Amazing Grace — Get the cultural background and meaning of the song.
By studying these, you’ll realize:
Music is just mathematics, and mathematics is perfectly codeable.
🎶 Basic Amazing Grace Melody Breakdown (C Major Key)
Let’s keep it simple in the C major scale (no sharps or flats).
Lyrics | Notes | Frequencies (Hz) |
---|---|---|
Amazing grace | C E G C | 261, 329, 392, 523 |
How sweet the sound | E G A G E C | 329, 392, 440, 392, 329, 261 |
That saved a wretch like me | E G A G E C | 329, 392, 440, 392, 329, 261 |
I once was lost | G A C E | 392, 440, 523, 329 |
But now am found | C E G E | 261, 329, 392, 329 |
Was blind but now I see | G A C | 392, 440, 523 |
(Note: some variations exist — we’ll use this simple one for coding.)
🛠️ How We’ll Represent It in Code
We’ll create two parallel lists:
- A list of frequencies
- A list of note durations (all around 400ms to 600ms depending on rhythm)
Example:
let frequencies = vec![261, 329, 392, 523, 329, 392, 440, 392, 329, 261, ...];
let durations = vec![400, 400, 400, 800, 400, 400, 400, 400, 400, 800, ...];
Later, we’ll loop through these lists,
playing each frequency for its corresponding duration.
🎯 Why Mapping Matters
When you map thoughts (music) into numbers (frequencies),
and numbers into action (sound waves),
you are literally coding your imagination into existence.
This process — mapping abstract beauty into pure logic —
is the essence of creative programming.
Concept | Summary |
---|---|
Notes are matched to frequencies (Hz) | ✅ |
Amazing Grace melody broken into simple sequences | ✅ |
Code plan prepared (frequency + duration vectors) | ✅ |
Ready to start coding the melody | ✅ |
✅ Now we have a full, clean blueprint for coding the Amazing Grace melody from scratch.
✅ Our next step is to start writing Rust code to play the first few notes!
🚀 Next Step
👉 Step 4: Rust Amazing Grace code tutorial: Writing the First Few Notes in Rust
📚 Step 4: Rust Amazing Grace code tutorial: Writing the First Few Notes in Rust
In this part of our Rust Amazing Grace code tutorial, we finally transform thought into sound.
Until now, we’ve prepared the canvas, gathered the colors, and drawn the blueprint.
Now, it’s time to pick up the brush and make music happen — one note at a time.
Our goal here is simple but powerful:
Take the first few notes of Amazing Grace and make them sing using pure Rust code.
🎼 Preparing the Notes and Durations
First, we need to define the notes (as frequencies) and their durations (in milliseconds).
Here’s the breakdown for the very first line of Amazing Grace (“Amazing grace”):
Lyrics | Note | Frequency (Hz) | Duration (ms) |
---|---|---|---|
A- | C4 | 261 | 500 |
-ma- | E4 | 329 | 500 |
-zing | G4 | 392 | 500 |
grace | C5 | 523 | 1000 |
- C4 = Middle C (261 Hz)
- E4 = E above middle C (329 Hz)
- G4 = G above middle C (392 Hz)
- C5 = High C (523 Hz)
We’ll store these values into two separate vectors:
one for frequencies, one for durations.
🛠️ Writing the Rust Code
Here’s how we turn those values into real sound:
use rodio::Source;
use std::{thread, time::Duration};
fn main() {
let device = rodio::default_output_device().expect("No audio output device available!");
// Define the sequence of frequencies (notes) and their durations
let frequencies = vec![261, 329, 392, 523]; // C4, E4, G4, C5
let durations = vec![500, 500, 500, 1000]; // milliseconds
for (freq, dur_ms) in frequencies.iter().zip(durations.iter()) {
let source = rodio::source::SineWave::new(*freq)
.take_duration(Duration::from_millis(*dur_ms as u64))
.amplify(0.20); // Lower volume to avoid distortion
rodio::play_raw(&device, source.convert_samples());
// Sleep to let the note finish before starting the next one
thread::sleep(Duration::from_millis(*dur_ms + 100)); // Slight pause between notes
}
}
🎶 What Happens Here?
- We define the sequence of notes (frequencies) and how long each note should play (durations).
- For each note:
- Create a sine wave with
SineWave::new(frequency)
. - Set how long it should play using
take_duration
. - Adjust the volume a bit lower with
amplify
. - Play it using
play_raw
. - Wait (
sleep
) long enough to finish the sound before moving to the next note.
- Create a sine wave with
✅ With this code, your speakers will now gently sing the opening of “Amazing Grace.”
🎯 Why This Matters
This isn’t just about hearing a song.
It’s about experiencing the pure act of creation:
- You thought about a melody.
- You mapped it into logic.
- You made it real — with nothing but code.
This direct translation from mind to reality is what builds mastery faster than anything else.
Task | Status |
---|---|
Frequencies and durations defined | ✅ |
Each note generated as a SineWave | ✅ |
Notes played in sequence with controlled timing | ✅ |
First part of Amazing Grace successfully coded | ✅ |
✅ Congratulations: you just made music out of raw thought.
✅ And you did it in Rust, using nothing but pure, elegant code. 🎶🦀
🚀 Next Step
👉 Step 5: Rust Amazing Grace code tutorial: Completing the Full Melody
📚 Step 5: Rust Amazing Grace code tutorial: Completing the Full Melody
You’ve already created the first few notes of Amazing Grace —
you’ve felt the magic of coding your thoughts directly into sound.
Now, in this stage of the Rust Amazing Grace code tutorial,
we take it further:
We complete the full melody.
This isn’t just about writing longer code.
It’s about maintaining momentum —
the critical skill that separates a casual learner from a true creator.
🎼 Full Melody Breakdown
Here’s a simple breakdown of the main part of Amazing Grace, staying in C Major:
Lyrics | Note | Frequency (Hz) | Duration (ms) |
---|---|---|---|
Amazing grace | C4 E4 G4 C5 | 261 329 392 523 | 500 500 500 1000 |
How sweet the sound | E4 G4 A4 G4 E4 C4 | 329 392 440 392 329 261 | 500 500 500 500 500 1000 |
That saved a wretch like me | E4 G4 A4 G4 E4 C4 | 329 392 440 392 329 261 | 500 500 500 500 500 1000 |
I once was lost | G4 A4 C5 E4 | 392 440 523 329 | 500 500 500 1000 |
But now am found | C4 E4 G4 E4 | 261 329 392 329 | 500 500 500 1000 |
Was blind but now I see | G4 A4 C5 | 392 440 523 | 500 500 1000 |
🛠️ Full Code to Play the Melody
Here’s how you put it all together:
use rodio::Source;
use std::{thread, time::Duration};
fn main() {
let device = rodio::default_output_device().expect("No audio output device available!");
// Frequencies of Amazing Grace in C Major
let frequencies = vec![
261, 329, 392, 523, // Amazing grace
329, 392, 440, 392, 329, 261, // How sweet the sound
329, 392, 440, 392, 329, 261, // That saved a wretch like me
392, 440, 523, 329, // I once was lost
261, 329, 392, 329, // But now am found
392, 440, 523 // Was blind but now I see
];
// Durations (ms) corresponding to each note
let durations = vec![
500, 500, 500, 1000,
500, 500, 500, 500, 500, 1000,
500, 500, 500, 500, 500, 1000,
500, 500, 500, 1000,
500, 500, 500, 1000,
500, 500, 1000
];
for (freq, dur_ms) in frequencies.iter().zip(durations.iter()) {
let source = rodio::source::SineWave::new(*freq)
.take_duration(Duration::from_millis(*dur_ms as u64))
.amplify(0.20);
rodio::play_raw(&device, source.convert_samples());
// Short pause between notes
thread::sleep(Duration::from_millis(*dur_ms + 100));
}
}
🎶 What Happens Here?
- The entire Amazing Grace melody plays through your speakers.
- Each note flows smoothly into the next.
- You’ve coded an entire song — not just a beep or a tone.
You’ve coded a feeling.
🎯 Why This Is Special
At this point, you’ve:
- Mapped a complete melody from mind to code
- Understood the structure of music on a numerical level
- Created a real song using nothing but math and Rust
This is powerful.
This is what turning ideas into reality feels like.
This is how creators are made.
Task | Status |
---|---|
Full Amazing Grace melody mapped | ✅ |
Frequencies and durations implemented | ✅ |
Melody successfully played in Rust | ✅ |
✅ Congratulations — you’ve fully coded Amazing Grace in Rust. 🎶🦀
✅ From inspiration → numbers → sound → reality.
🚀 Next Step
👉 Step 6: Rust Amazing Grace code tutorial: Fine-Tuning the Sound
📚 Step 6: Rust Amazing Grace code tutorial: Fine-Tuning the Sound
You’ve now fully coded the entire melody of Amazing Grace in Rust.
You’ve proven you can turn pure thought into music.
But — as any true artist knows —
it’s not just about creating.
It’s about refining.
In this part of our Rust Amazing Grace code tutorial, we’ll make the sound more polished and beautiful.
Small tweaks will turn “basic” into “professional.”
🎶 Why Fine-Tuning Matters
Without fine-tuning, your melody can sound:
- Mechanical
- Rushed
- Harsh or too loud
Tiny adjustments — in volume, pacing, and silence — can make the music breathe, making it feel human and emotional.
Just like in coding:
Clean output is the result of careful, thoughtful fine-tuning.
🛠️ Fine-Tuning Techniques
Here’s what we’ll improve:
1. Adjusting Volume Smoothly
Currently, every note plays at 20% of the full volume (amplify(0.20)
).
This is good, but you can try dynamically adjusting volume based on musical phrasing.
Example idea:
- Play higher notes slightly softer.
- Keep emotional peaks at a stronger volume.
(For now, we can just slightly soften everything a bit more to make it gentler.)
.amplify(0.15) // instead of 0.20
2. Adding Slight Pauses for Breathing Room
Currently, after each note, we wait:
thread::sleep(Duration::from_millis(*dur_ms + 100));
100ms pause is good,
but for longer notes (like 1000ms notes), we can make the pause slightly longer.
Idea:
let pause = if *dur_ms > 700 { 300 } else { 100 };
thread::sleep(Duration::from_millis(*dur_ms + pause));
- Longer notes → Longer breathing space
- Shorter notes → Shorter breathing space
This makes the flow feel more natural.
3. Adding a Fade-Out Effect (Advanced)
If you want to make notes fade out softly instead of ending abruptly,
you could build a custom Source that fades the volume at the end of each note.
But to keep it simple now:
- We’ll soften the attack/decay by adjusting the amplifier very slightly.
(e.g., lower amplify
overall.)
✨ Updated Code Snippet (Fine-Tuned)
use rodio::Source;
use std::{thread, time::Duration};
fn main() {
let device = rodio::default_output_device().expect("No audio output device available!");
let frequencies = vec![
261, 329, 392, 523,
329, 392, 440, 392, 329, 261,
329, 392, 440, 392, 329, 261,
392, 440, 523, 329,
261, 329, 392, 329,
392, 440, 523
];
let durations = vec![
500, 500, 500, 1000,
500, 500, 500, 500, 500, 1000,
500, 500, 500, 500, 500, 1000,
500, 500, 500, 1000,
500, 500, 500, 1000,
500, 500, 1000
];
for (freq, dur_ms) in frequencies.iter().zip(durations.iter()) {
let source = rodio::source::SineWave::new(*freq)
.take_duration(Duration::from_millis(*dur_ms as u64))
.amplify(0.15); // Slightly softer for a smoother feel
rodio::play_raw(&device, source.convert_samples());
// Dynamic pause based on note length
let pause = if *dur_ms > 700 { 300 } else { 100 };
thread::sleep(Duration::from_millis(*dur_ms + pause));
}
}
🎯 What This Fine-Tuning Achieves
- Notes feel more natural and emotional.
- Flow between notes is smoother.
- Overall sound feels gentler, less harsh.
✅ It’s a subtle change — but it’s the kind of subtlety that turns a project from “cool” to “beautiful.”
Task | Status |
---|---|
Adjusted overall volume | ✅ |
Dynamic pauses between notes | ✅ |
Sound flow refined | ✅ |
✅ Now, you haven’t just coded Amazing Grace —
✅ You’ve crafted it.
✅ Your melody now breathes like real music.
🚀 Next Step
👉 Step 7: Rust Amazing Grace code tutorial: Reflecting on What We Built
📚 Step 7: Rust Amazing Grace code tutorial: Reflecting on What We Built
In this final part of the Rust Amazing Grace code tutorial,
we step back —
and look at what we’ve really done.
It’s not just about coding sound.
It’s not just about making music.
It’s about proving a truth:
If you act immediately on your ideas,
you will grow.
🎶 What We Actually Achieved
Think about where we started:
- An idea: “Can I make Amazing Grace with code?”
- A spark: “What if I tried right now?”
- An action: “Open Rust. Start typing.”
No waiting.
No second-guessing.
Just moving — thought into action, imagination into code, code into music.
✅ You learned how to set up a Rust sound project.
✅ You understood how sound is just pure math.
✅ You broke down an entire melody into codeable units.
✅ You built, refined, and played it.
✅ You experienced the flow from thought → code → reality.
🧠 Why Immediate Coding Changes You
Most people lose their ideas.
They think: “I’ll remember it later.”
They plan. They prepare. They delay.
But true creators — real developers —
act immediately.
They catch the spark when it’s fresh.
They code when the feeling is alive.
They build while the dream is burning inside them.
Because thoughts decay.
Inspiration has a half-life.
The faster you move, the more you capture.
The more you capture, the faster you grow.
🎯 Your New Power
Through this small but beautiful project,
you’ve trained a priceless reflex:
See it → Code it.
Think it → Make it.
Hear it → Play it.
And every time you repeat this cycle,
you get closer to mastery.
Key Principle | Insight |
---|---|
Thinking is not enough. | Action is what transforms ideas. |
Acting fast captures inspiration. | Waiting kills momentum. |
Immediate coding builds real skill. | Coding directly from thought is the fastest way to grow. |
✅ You didn’t just follow a tutorial.
✅ You practiced a way of thinking — a way of creating — that will accelerate your journey forever.
🎶 Final Words
From a single fleeting idea,
you built Amazing Grace with nothing but math, code, and determination.
Imagine what else you can build now.
Imagine how far you can go
if you simply keep chasing your ideas — immediately, relentlessly, fearlessly.
🚀 What’s Next?
What will you code the next time a thought sparks in your mind?
Don’t wait.
Catch it.
Build it.
Make it real.
Because now you know how.
🎉 Congratulations — you’re no longer just a coder.
You’re a creator.