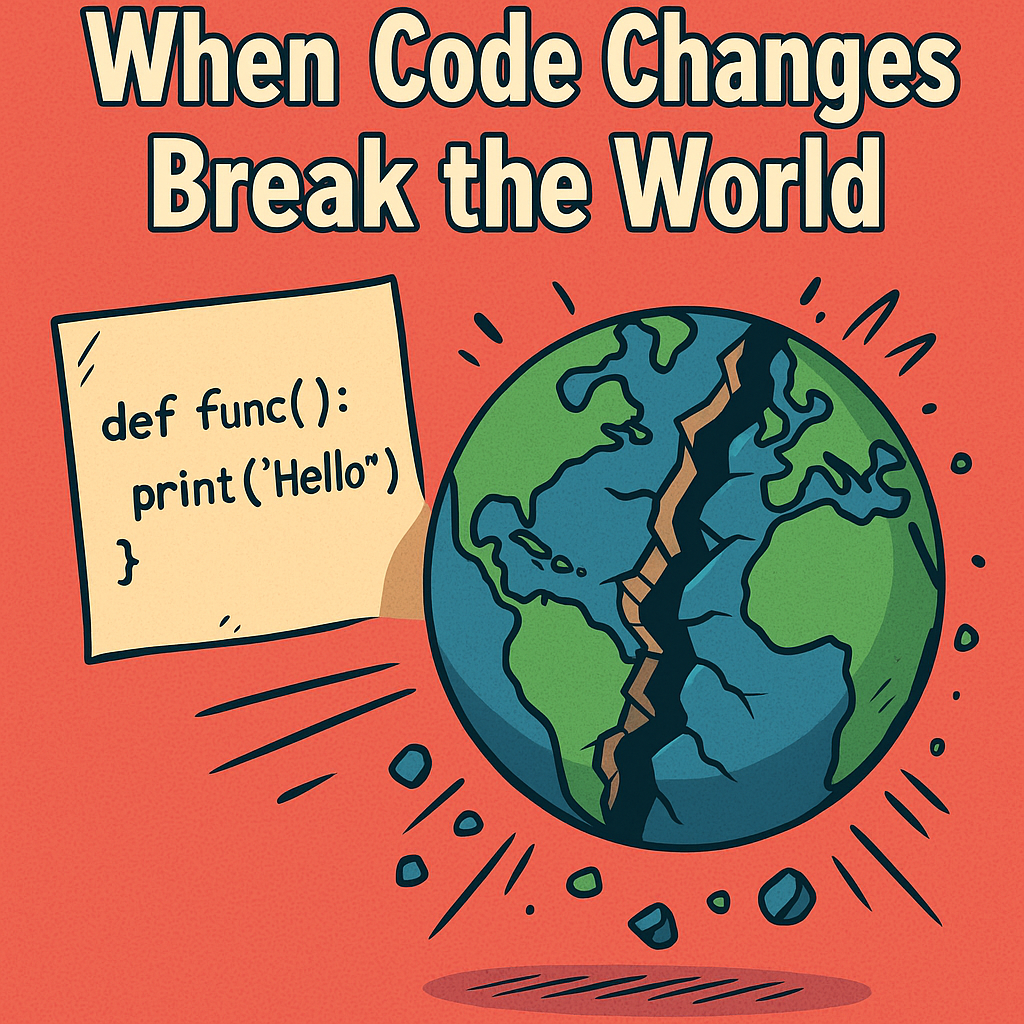
🔧 1. Why Python Backward Compatibility Matters When Code Suddenly Breaks
To be completely honest, I’m not a fan of Python backward compatibility.
That might sound dramatic, but after spending countless hours debugging code that used to work just fine — only to find out it broke due to a seemingly minor version change — I’ve developed a bit of a grudge.
There’s nothing quite like pulling a snippet from an official-looking tutorial or GitHub repo, running it, and watching it fail for reasons that aren’t obvious. Then comes the rabbit hole: StackOverflow threads, changelogs, version checks, and finally the realization — “Oh, this worked in Python 3.7, but I’m on 3.10.”
🤯 Why It Feels So Frustrating
Python markets itself as beginner-friendly and developer-centric. And in many ways, it absolutely is.
But backward compatibility? Not so much.
- Function signatures change quietly
- Modules get shuffled around or deprecated
- Built-ins evolve or disappear
- Type hinting suddenly behaves differently
- And worst of all: code looks fine, but behaves differently across versions
It’s like walking across a familiar room in the dark, only to stub your toe on a piece of furniture someone moved without telling you.
🧠 But Here’s the Thing: It Matters
Despite my personal frustrations, Python backward compatibility is more than just a nuisance — it’s a vital (and complex) part of the language’s evolution.
The Python community intentionally prioritizes progress over absolute backward safety. Unlike some languages that try never to break things (and become bloated as a result), Python takes the opposite stance: “If it’s bad design, let’s fix it — even if it breaks things.”
That mindset has kept Python clean, modern, and expressive — but at a cost.
💡 The Developer’s Reality
If you’ve ever:
- Watched a working project break after a pip upgrade,
- Struggled to replicate behavior between your machine and a teammate’s,
- Or chased mysterious bugs only to find a subtle version difference…
You’ve lived through the chaos that poor backward compatibility can cause.
And while some embrace Python’s fluidity, others — like me — approach every version upgrade with a healthy dose of anxiety.
Table of Contents
🔄 2. How Python Backward Compatibility Struggles with Version Evolution
Python is constantly improving — and I love that.
New syntax, cleaner APIs, better performance — all good things. But every time I hear “Python 3.X is out!”, a part of me winces. Because with every step forward, I’ve learned to expect at least two steps backward in compatibility.
⚙️ Progress vs. Compatibility: The Eternal Trade-off
Let’s face it: you can’t evolve a language for 30+ years without breaking a few things.
The tricky part is that Python doesn’t try to avoid breaking things — it embraces it.
Take Python 3’s release, for example:
print
became a function — cleaner, yes, but broke almost every 2.x script.- String handling shifted to Unicode by default — essential for global apps, but a nightmare for old codebases.
- Division behavior changed — because math should make sense, but legacy logic often depended on the old way.
Each of these changes was justified. Each of them made the language better.
But they all came at a cost: backward compatibility suffered.
🧬 Breaking for the Sake of Clarity
Python’s “Zen” says it best: “There should be one– and preferably only one –obvious way to do it.”
To get there, sometimes the old ways have to go. This means:
- Deprecated methods disappear
- Legacy behavior gets replaced
- Ambiguous syntax is cleaned up
While this improves the language for newcomers and future development, it often feels like the past is being erased — and anyone maintaining older code is left to pick up the pieces.
🧠 Why It’s Not Just a Python Problem
To be fair, Python isn’t the only language that faces this.
JavaScript went through similar chaos moving from ES5 to ES6. Even Java, known for being ultra-stable, has deprecated APIs that frustrate enterprise developers.
But here’s the difference: Python changes fast. And its community encourages those changes.
This agility is part of what makes Python modern — but it also makes version fragmentation a constant headache.
🚧 So What Can We Learn?
Version evolution is necessary — even exciting.
But for developers, every upgrade requires caution. Every change in version means asking:
- What just got deprecated?
- Will this library still work?
- Is this tutorial still valid?
- Do I really need to upgrade right now?
In a world where codebases age in dog years, Python backward compatibility isn’t just a technical issue — it’s a survival strategy.
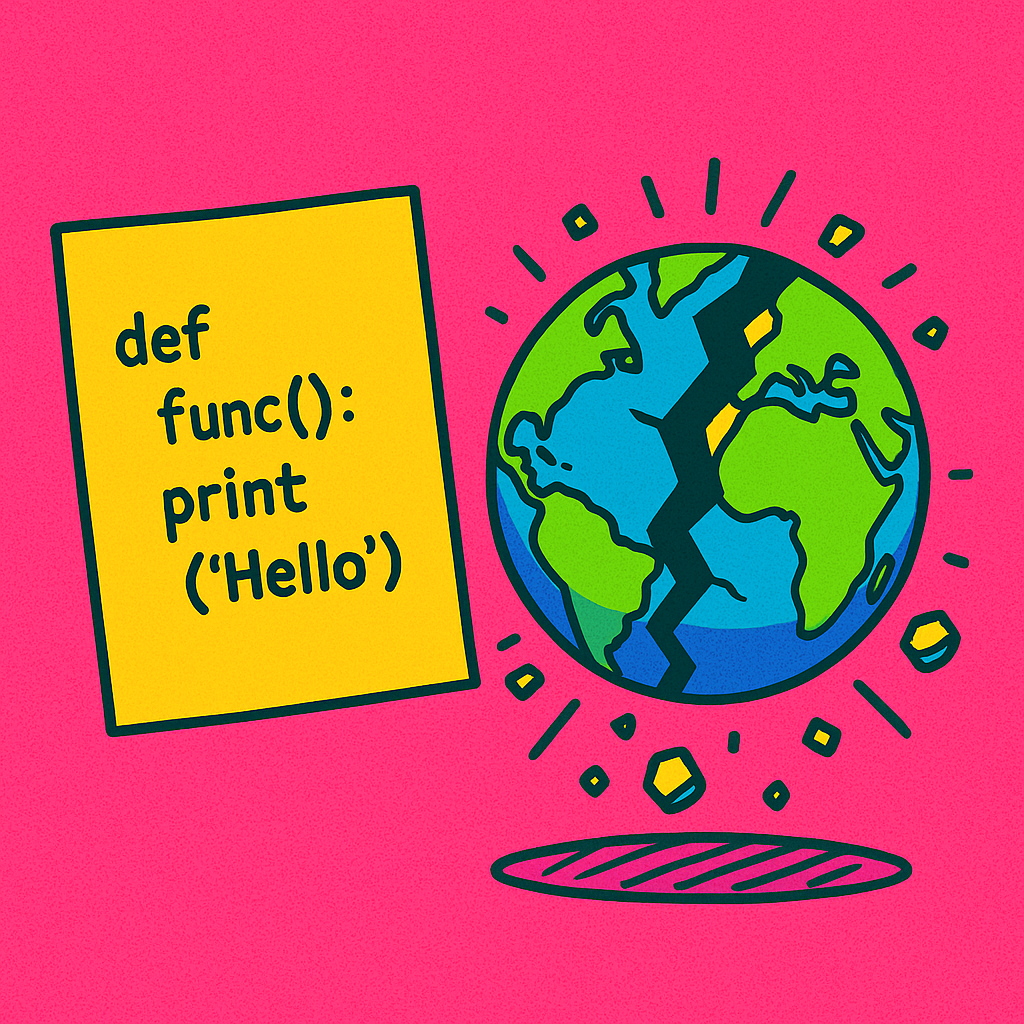
📦 3. The Hidden Risks of Python Backward Compatibility in Standard Modules
One of the more deceptive aspects of Python is how easy it seems — until it isn’t.
You find a solution on Stack Overflow, copy a few lines of code, and run it with confidence. And then — boom — AttributeError
, ImportError
, or worse, the code runs but produces a subtly wrong result. That’s when you realize: you’re not just dealing with Python — you’re dealing with Python and its version-specific behavior.
This is one of the most underappreciated dangers of Python backward compatibility: even core modules in the standard library evolve over time, and those changes can silently impact your code.
🧱 Case Study 1: asyncio
— Same Name, New Behavior
Take asyncio
, Python’s asynchronous I/O library. Between Python 3.6 and 3.10, the way you run an event loop changed significantly.
In Python 3.6:
python복사loop = asyncio.get_event_loop()
loop.run_until_complete(my_coroutine())
In Python 3.7+:
python복사asyncio.run(my_coroutine())
If you’re following a modern tutorial while stuck on an older Python version, that innocent line of code (asyncio.run
) simply doesn’t exist. No warnings. Just an AttributeError
.
Now imagine a junior developer trying to learn async programming and hitting this wall on day one.
That’s not just frustrating — it’s discouraging.
🧪 Case Study 2: typing
and the Moving Target of Type Hints
Python’s type hinting ecosystem is another example of backward compatibility pain.
The typing
module has evolved aggressively:
TypedDict
,Literal
,Final
, and others were introduced gradually across versions 3.5 to 3.10.- In early versions, they didn’t exist — or had to be imported from
typing_extensions
.
What does that mean in practice?
A library using modern type hints may not install correctly (or even parse) in slightly older environments.
Developers building tooling around type checking must constantly check version constraints and provide fallbacks.
🔁 Deprecated Today, Gone Tomorrow
Python often introduces changes with a grace period — functions are marked as “deprecated,” and only later removed entirely. But even during that transition:
- Warnings can be silenced or ignored
- Behavior may shift subtly
- Documentation might not be updated fast enough
The collections
module offers a great example. In Python 3.10:
python복사from collections import MutableMapping # Deprecated
In Python 3.10+, you should use:
python복사from collections.abc import MutableMapping
That’s a small change — but for automated tools, static analyzers, and cross-version scripts, it adds up. Multiply this by dozens of such changes, and suddenly you’re managing a compatibility matrix you never asked for.
📎 The Psychological Cost of “Almost Working”
What makes these risks so hard to manage is that they don’t always result in fatal errors.
Sometimes the code:
- Works in development, fails in production
- Behaves inconsistently across OS platforms
- Only fails under certain inputs
These “almost working” scenarios are often worse than total failures — because they erode trust. Developers start to second-guess every module import, every syntax pattern, every third-party dependency.
It becomes mentally exhausting to wonder: Is this a bug in my code — or just Python being Python across versions?
🧠 Why This Matters for Every Python Developer
Python’s standard library is one of its greatest strengths — but it’s also a moving target.
If you’re building tools, libraries, or services that need to survive across multiple Python versions, you need to actively track these changes, test across versions, and sometimes even maintain compatibility layers yourself.
In theory, backward compatibility should protect you from this. In practice, Python backward compatibility doesn’t always extend to behavior or module-level details.
The language itself is stable enough. But the ecosystem is fluid, and that’s where most real-world breakage happens.
💥 4. Common Real-World Pitfalls Caused by Python Backward Compatibility Issues
If you’ve ever screamed at a CI pipeline, stared blankly at a Docker log, or wondered why your teammate’s machine runs the exact same script without a hitch — you’ve likely felt the sting of Python backward compatibility in the wild.
And let’s be honest: these issues don’t happen in toy projects.
They strike at the heart of real software — production APIs, ML pipelines, internal tools, deployment scripts.
And they never show up at a convenient time.
🚨 Pitfall #1: The Dev vs. Prod Version Clash
It starts innocently.
You’re developing on Python 3.9, but your production server is still running 3.7. You finish your feature, everything works locally, CI passes… then deployment happens. Boom. SyntaxError
.
Why? You used:
python복사def greet(name: str | None) -> str:
The PEP 604 syntax for str | None
only works in Python 3.10+.
On your machine: fine. On prod: crash.
This is perhaps the most insidious form of backward compatibility failure: invisible local success → remote disaster.
🧩 Pitfall #2: Dependency Hell (a.k.a. “The Requirements Trap”)
Let’s say you depend on:
requests==2.31.0
pandas==2.1.0
boto3
All of them work — individually. But pandas
now requires Python 3.9+, and your production base image uses Python 3.7.
Now you’re trapped:
- Upgrade Python and break other legacy systems?
- Downgrade pandas and lose new features?
- Rewrite everything just to dodge a version issue?
You’ve now entered the world of dependency paralysis, and Python backward compatibility just became a full-blown architectural problem.
🧪 Pitfall #3: Test Suites That Lie
You write unit tests. They pass locally.
They pass in CI.
They pass in staging.
But one user reports a crash. Another sees incorrect output. The third? Silent failure.
Turns out the bug only triggers in Python 3.8 on Windows, when a particular optional dependency isn’t installed. Your test matrix? It only covered Linux + 3.10.
This is where Python backward compatibility bites hard — because it’s not just about syntax, it’s about:
- OS-specific behavior
- Package version conflicts
- Deprecated APIs still silently in use
🧱 Pitfall #4: Legacy Code That Won’t Die
Most teams carry some technical debt — that one internal CLI tool written in 2017, or the dusty ETL script that somehow still runs every night.
And guess what? That code:
- Relies on
collections.MutableMapping
- Imports
imp
instead ofimportlib
- Has raw string comparisons that break with Unicode
Now your team wants to upgrade to Python 3.11. Good luck.
Suddenly, you’re not writing features anymore — you’re doing archaeological compatibility refactoring, just to keep things from falling apart.
😫 Why These Pitfalls Hurt So Much
These aren’t beginner problems. These are senior-dev-on-a-deadline-trying-to-push-to-prod problems.
What makes them so painful is their unpredictability. One team might go years without a problem — then a single system update unearths a compatibility landmine. And in large orgs, with multiple Python versions in use across microservices, automation, data pipelines… it becomes unmanageable fast.
Python’s flexibility is a gift — but backward compatibility is where that flexibility turns into fragility.
🧠 Takeaway: Expect Breakage. Plan for It.
There’s no silver bullet. But if you accept that Python backward compatibility is always a factor, you can prepare for it:
- Pin your dependencies
- Test across multiple versions
- Use tools like
tox
,pyenv
, and CI matrices - Write migration-friendly code
Because in the real world, “works on my machine” isn’t enough — not when Python keeps changing the rules behind the scenes.
🛠️ 5. When Breaking Python Backward Compatibility Is the Right Choice
So far, we’ve ranted.
We’ve debugged.
We’ve suffered.
But now it’s time to ask the harder question:
What if breaking Python backward compatibility… is actually a good thing?
I know — it feels wrong even saying it out loud. But there’s a reason Python occasionally throws backward compatibility out the window. And in many cases, it’s not just justified — it’s absolutely necessary.
🧨 Why Would Anyone Intentionally Break Compatibility?
The answer is simple: bad code has to die.
Not your code, of course. But old, inconsistent, confusing patterns that have lingered for years — because nobody wanted to clean them up.
Python, to its credit, has a clear philosophy. The Zen of Python doesn’t say “never change.”
It says: “Now is better than never. Although never is often better than right now.”
Translation? “Let’s fix it… but let’s do it with care.”
Sometimes, fixing a language means pulling weeds — even if it means your garden looks messy for a while.
🧱 Examples of Breaking That Made Python Better
Here are just a few controversial compatibility breaks that, in hindsight, improved the language:
✅ print
as a function (Python 3)
python복사# Old (2.x)
print "Hello"
# New (3.x)
print("Hello")
Why it was worth it:
- More consistent syntax
- Easier to pass as a first-class object
- Aligns with other programming languages
✅ Unicode by default
Python 2’s string model was a global mess.
Python 3 made str
unicode by default — a massive change, but essential for global applications.
✅ Division behavior
python복사# Python 2
5 / 2 # → 2
# Python 3
5 / 2 # → 2.5
Was it painful? Yes.
Was it mathematically correct? Also yes.
Each of these changes broke thousands of scripts. But they also cleaned up core inconsistencies and set Python on a better path.
🔥 The Cost of Eternal Compatibility
Let’s imagine the alternative.
What if Python kept every odd behavior just to maintain compatibility?
- Legacy string encoding quirks would live on forever.
- Insecure or misleading functions would stay “for backward compatibility.”
- New features would have to tiptoe around landmines left behind by old syntax.
Eventually, the language would become bloated, brittle, and incoherent — a museum of bad decisions, preserved for fear of breaking someone’s code.
In other words: backward compatibility at all costs leads to stagnation.
📈 Python’s Strategy: Break Smart, Warn Early
Python does break compatibility — but it rarely breaks trust. Here’s how:
- New behavior is proposed and discussed publicly via PEPs (Python Enhancement Proposals)
- Changes are announced well in advance
- Deprecation warnings are added to alert developers before removal
- Tools like
2to3
andpyupgrade
help automate migration
Python’s goal isn’t chaos — it’s clarity. And sometimes, clarity demands a reset.
🧠 What It Means for Developers
It’s easy to complain about changes — especially when they break your code.
But Python’s willingness to evolve is part of what keeps it relevant.
As a developer, the key isn’t to fight every change. It’s to:
- Understand why the break happened
- Follow deprecation cycles
- Design your code to be adaptable
After all, if we demand that Python never break anything…
We might end up with a language that’s safe, but stuck in the past.
💬 TL;DR
Yes, backward compatibility is important.
But no, it shouldn’t be sacred.
Sometimes breaking things is how Python gets better — even if it means a little short-term pain.
And honestly? I respect that.
📜 6. How the Community Manages Python Backward Compatibility Through PEPs and Deprecation
For a language that breaks things as often as Python, you’d think it would feel more… chaotic.
But strangely, it doesn’t.
And that’s because Python backward compatibility isn’t broken randomly — it’s managed. Carefully. Transparently. Sometimes frustratingly slowly. But it’s managed.
At the heart of that process is one thing: PEPs — Python Enhancement Proposals.
📌 What’s a PEP, and Why Should You Care?
A PEP is a formal document that proposes a change to Python. It can be about:
- A new syntax (
match
statements? → PEP 634) - A new module (
dataclasses
? → PEP 557) - A backwards-incompatible change (like removing
asyncio.Task.all_tasks()
? → PEP 585 follow-up)
And every single meaningful change to Python — especially those that break backward compatibility — goes through this process.
Why this matters:
- You can read them. Right now. For free.
- You can see community discussion and dissent.
- You get advance warning about what’s coming.
PEPs are like the changelogs of Python’s soul.
🔔 Deprecation: The Gentle Way of Saying “This Will Die”
Before anything is removed, Python tries to give you a heads-up. That’s where deprecation comes in.
When something is deprecated:
- It still works (for now)
- But using it triggers a warning (sometimes only in verbose/test mode)
- And it’s flagged for future removal in documentation
This gives developers time to adapt, tools to scan their code, and enough lead time to plan upgrades.
For example, collections.MutableMapping
was used everywhere — until Python 3.10 deprecated it in favor of collections.abc
. It wasn’t removed right away.
Instead, it was part of a multi-year transition. That’s empathy.
🧪 The Role of Tooling in Compatibility Management
Python doesn’t just rely on humans reading PEPs. It also provides tools and patterns that help you:
warnings
module → catch deprecation notices in runtimetox
,nox
→ test your code across multiple Python versionspyupgrade
,2to3
,modernize
→ auto-refactor code for newer versions- Linters and static analyzers → flag deprecated usage before it becomes an error
These tools form a kind of compatibility safety net, ensuring that you’re never totally caught off guard.
🧠 Why This Process Actually Works
The result of all this structure?
Even when Python breaks backward compatibility, it rarely feels like betrayal.
That’s because:
- You see it coming
- You understand why
- You have time to adjust
- And you have tools to help
Sure, it’s still annoying. But it’s also honest. Transparent change is easier to accept — even if it stings.
Contrast that with ecosystems where things break without warning, or where new versions are pushed silently with breaking changes buried in a blog post.
Python, by comparison, is practically gentle.
⚖️ The Balancing Act: Stability vs. Sanity
Maintaining full backward compatibility forever would paralyze the language.
Breaking everything all the time would alienate the community.
So Python walks a tightrope — and the PEP + deprecation + tooling process is how it balances.
It’s not perfect. But it’s a hell of a lot better than chaos.
💬 TL;DR
Python doesn’t break your code out of spite.
It breaks it because it’s trying to get better — and it tells you how, when, and why.
Between PEPs, deprecation warnings, and powerful tooling, Python backward compatibility is not just managed — it’s governed.
That might not make the breakages feel good…
But it does make them fair.
🛡️ 7. Developer Survival Tips for Handling Python Backward Compatibility Problems
Let’s face it — you can’t stop Python from changing.
But you can prepare for it.
If you’ve ever had a production system crash, a CI pipeline implode, or your laptop scream at a SyntaxError
because of a version mismatch, this section is for you.
Here are the battle-tested, caffeine-fueled survival strategies I’ve learned (often the hard way) for dealing with Python backward compatibility issues — without losing your sanity.
🔐 1. Pin Everything — Yes, Everything
Let’s start with the basics.
Never — and I mean never — leave your requirements.txt
looking like this:
nginx복사flask
numpy
pandas
Why? Because if you install these tomorrow, or on another machine, you’re not getting the same environment — you’re getting whatever the latest versions are today. And that might mean:
- New bugs
- Deprecated functions
- Subtle behavioral changes
What to do instead:
- Use
pip freeze
to lock exact versions - Commit your
requirements.txt
orpyproject.toml
to source control - Use
pip-tools
,poetry
, orpipenv
for dependency management
Pinned dependencies = predictable behavior. Simple as that.
🔁 2. Test Across Python Versions (Before It’s Too Late)
It’s easy to write code that works on your version of Python.
The challenge? Making sure it works on the versions your users or teammates have.
Use tools like:
tox
– test your code in isolated environments with different Python versions- GitHub Actions – add a test matrix:
3.7
,3.8
,3.9
,3.10
,3.11
- Docker – build repeatable images with specific versions baked in
It might feel like overkill, but I promise — the day someone runs your CLI tool in Python 3.7 and it explodes, you’ll wish you had a test matrix.
🧪 3. Watch for Deprecation Warnings (Don’t Just Ignore Them!)
Python is polite. It warns you when something is going away.
But if your test runner suppresses warnings — or you ignore them — you’re walking into a trap.
What to do:
- Run tests with
-Wd
to surface all warnings - Use
pytest
‘s--strict-markers
and warning filters - Actively remove deprecated code before it becomes a hard failure
Think of warnings as Python saying:
“Hey… I’m changing this. You might wanna fix that.”
🧰 4. Use Compatibility Tools and Patterns
Python has an amazing ecosystem of helpers for bridging versions:
six
– for writing Python 2/3 compatible code (less common now, but still useful in legacy projects)typing_extensions
– backports of modern type features to older Python versionsdataclasses
backport – for pre-3.7 environmentsfuture
,__future__
– enable newer syntax in older versions
And don’t be afraid to use version checks in your code:
python복사import sys
if sys.version_info < (3, 8):
# fallback logic
else:
# use modern API
It’s not “clean,” but neither is broken software.
📦 5. Isolate Environments Like Your Life Depends on It
Because sometimes, it does.
Don’t develop in your system Python. Use:
virtualenv
orvenv
conda
if you’re in data science landpyenv
to install and switch between multiple Python versions
Each project gets its own isolated environment. No overlap. No weird conflicts.
Just peace of mind.
🧠 6. Think Forward, Write Backward-Compatible
When writing new code:
- Avoid version-specific syntax unless necessary
- Don’t use bleeding-edge features in critical tools
- Keep an eye on PEP status updates if you’re planning long-term support
If you maintain open source, support at least two Python versions if you can.
If you’re in a company? Push for language version alignment across teams.
🔍 7. Audit and Monitor Regularly
You’re not done once it “works.” Stay proactive:
- Run
safety
orbandit
to check for insecure/outdated packages - Use Dependabot or Renovate to get alerts for new versions
- Scan your codebase for deprecated usage every few months
Treat compatibility like you would security — not something to “fix later,” but something to manage continuously.
💡 Final Thought: It’s Not About Being Perfect — Just Prepared
Python backward compatibility issues will happen.
But if you plan for them, test for them, and isolate your environments, they don’t have to become disasters.
The truth is, being a Python developer today means being a compatibility manager, too.
It’s not glamorous — but it’s what keeps your software alive.
🌱 8. Looking Beyond: How Python’s Compatibility Pains Are Fueling the Rise of New Languages Like Mojo
After years of navigating Python’s growing ecosystem — along with the inevitable challenges of backward compatibility — a natural question arises:
Is it time to try something new?
Python is incredibly powerful, but it’s also been around for decades. And with that history comes legacy. While we love its flexibility, friendliness, and libraries, many developers are starting to wonder:
Can we have Python’s strengths without its limitations?
One of the most compelling answers right now is Mojo — a new language that blends the feel of Python with the performance of systems programming.
⚡ What Is Mojo, and Why Is It Gaining Attention?
Mojo is a next-generation programming language built by the team at Modular. It aims to combine:
- Python-like syntax
- The raw performance of C++
- Safety and concurrency similar to Rust
- Seamless integration with the Python ecosystem
It’s designed from the ground up to solve the problems Python was never meant to tackle — especially in AI, data-intensive workloads, and systems-level programming — without carrying decades of legacy baggage.
And perhaps most appealing of all?
You can use Python libraries in Mojo, and write high-performance code using a language that looks and feels familiar.
🧭 Getting Started with Mojo on Windows
If you’re curious and ready to give Mojo a spin, I’ve written a complete hands-on guide for setting up Mojo on Windows:
👉 Set Up a Mojo Development Environment on Windows
In that article, you’ll learn:
- How to install the Mojo SDK and CLI
- How to configure your environment correctly
- How to run and test basic Mojo code
This will give you everything you need to start exploring Mojo in your own projects — especially if you’re coming from a Python background.
📂 Mojo’s Official GitHub Repository
If you’d like to dive directly into the source, examples, and latest updates, you can check out the official Mojo GitHub repository here:
🔗 Mojo GitHub – github.com/modularml/mojo
There, you’ll find:
- Language samples
- Compiler updates
- Community discussions
- Contribution guidelines
🧠 Final Thoughts: Beyond Compatibility, Toward Possibility
Python isn’t going away — nor should it.
But the rise of Mojo signals something deeper: developers want evolution without the baggage.
Where Python gave us flexibility, Mojo offers performance.
Where Python gave us reach, Mojo adds precision.
Maybe it’s not about replacing Python — maybe it’s about expanding what’s possible with a Pythonic mindset.
If Python backward compatibility has ever made you hesitate, maybe it’s time to explore the next step — not to abandon what works, but to build on it with something stronger.