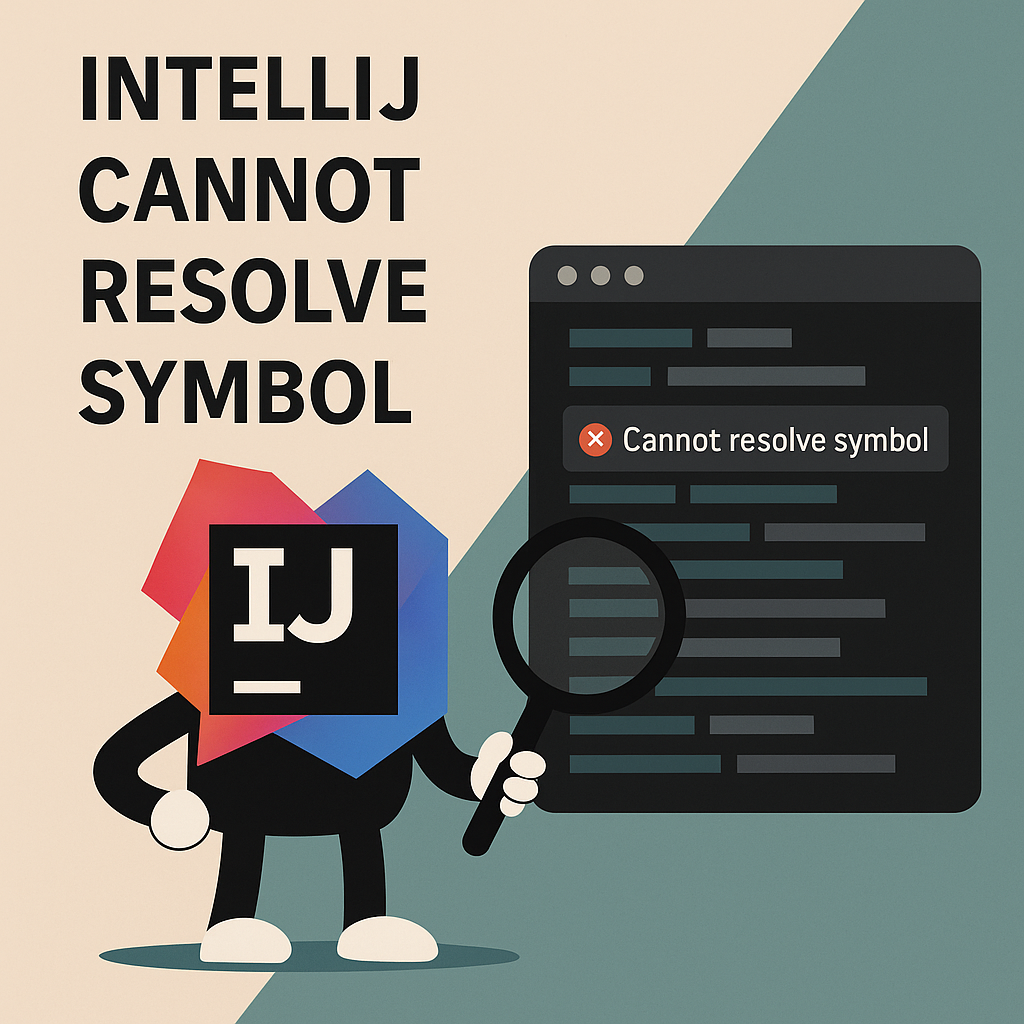
đź§© Section 1: Why IntelliJ Cannot Resolve Symbol Happens
If you’re seeing the “IntelliJ cannot resolve symbol” error, you’re not alone. This common issue frustrates many developers, especially when the symbol (class, method, or variable) clearly exists in the code. But what exactly causes it?
🔍 Common Causes of the “IntelliJ Cannot Resolve Symbol” Error
- IDE not synced with build tool (Gradle/Maven)
IntelliJ might not be aware of recently added dependencies, leading to missing symbols. - Incorrect or missing SDK configuration
If your project SDK is outdated or missing, IntelliJ will fail to resolve basic Java classes. - Corrupted cache or indexing issues
Internal IntelliJ caches may become outdated or broken, especially after updates or refactors. - Broken module/classpath structure
Your source files might not be marked correctly, or module dependencies may be missing. - Annotation processor not enabled (e.g., Lombok)
If you’re using tools like Lombok and don’t enable annotation processing, IntelliJ cannot resolve generated methods or constructors.
đź’ˇ What the Error Message Really Means
The “IntelliJ cannot resolve symbol” message usually appears:
- In the editor (red underline)
- As a tooltip when you hover over the missing symbol
- In the Problems tool window
This means that IntelliJ IDEA doesn’t know where to find the definition of the symbol, even if it’s actually present in your codebase. It’s an IDE-level issue—not necessarily a Java compiler problem.
đź§ Pro Tip
You can often fix this error without changing your code. Instead, you’ll need to adjust project settings, rebuild caches, or reimport dependencies. This guide will walk you through 7 reliable ways to fix IntelliJ cannot resolve symbol errors in the following sections.
🔄 Section 2: Sync Gradle or Maven to Fix IntelliJ Cannot Resolve Symbol
One of the most common causes of the IntelliJ cannot resolve symbol error is a mismatch between your IDE and your build system. If you’re using Gradle or Maven, and IntelliJ hasn’t fully imported the dependencies, you’ll likely run into missing classes or packages—even when they exist in your configuration files.
đź› Why Gradle or Maven Sync Matters
When IntelliJ doesn’t detect external libraries or modules defined in your build.gradle
or pom.xml
, it will throw IntelliJ symbol error messages. The IDE simply doesn’t know that those classes exist until you refresh the project structure.
âś… How to Fix It
For Gradle:
- Open the Gradle tool window (usually on the right).
- Click the Refresh icon (🔄) to sync the project.
- Alternatively, use the menu:
File > Sync Project with Gradle Files
For Maven:
- Open the Maven Projects tool window.
- Click Reimport All Maven Projects.
- You can also use
File > Invalidate Caches / Restart...
to reset everything if syncing fails.
🔍 Common Clues That a Sync Is Needed
- IntelliJ marks common libraries like
List
,Scanner
, orSpringApplication
as unresolved. Cannot resolve symbol 'X'
shows up even though the dependency is listed inbuild.gradle
orpom.xml
.- Your project builds fine via CLI (
./gradlew build
ormvn clean install
), but fails in the IDE.
đź’ˇ Bonus Tip
You can enable auto-import in IntelliJ to avoid this problem in the future:
- Go to
File > Settings > Build, Execution, Deployment > Build Tools > Gradle
- Check “Auto-import”
This keeps your project synced and avoids unnecessary IntelliJ resolve issues.
⚙️ Section 3: Check Project SDK and Language Level in IntelliJ
If your project’s SDK or language level isn’t set correctly, you’ll often encounter the IntelliJ cannot resolve symbol error—especially with standard Java classes like Scanner
, List
, or even System
.
🔍 Why SDK Configuration Causes Symbol Errors
IntelliJ relies on the Project SDK to understand the core Java libraries. If no SDK is set, or if it’s incompatible with your code, IntelliJ may fail to recognize even basic Java symbols. This leads to misleading errors such as:
Cannot resolve symbol 'Scanner'
Cannot resolve symbol 'List'
Even though these classes are part of the Java Standard Library, without a valid SDK, IntelliJ treats them as undefined.
âś… How to Fix It
Step-by-step:
- Go to
File > Project Structure > Project
- Make sure Project SDK is set (e.g.,
Java 17
,Java 21
, etc.)- If not, click “New…” and point to your JDK installation folder.
- Under Project language level, select the level that matches your code (e.g.,
17 - Sealed types
,11 - Local variable syntax for lambda parameters
, etc.)
Pro tip:
- You can also set Module SDK under
Project Structure > Modules > Dependencies
- This is useful when working with multi-module projects
đź’ˇ Quick Verification
To confirm if SDK is working:
- Try importing
java.util.List
orjava.util.Scanner
- If IntelliJ still shows
IntelliJ cannot resolve symbol
after setting the SDK, invalidate caches (see Section 5)
⚠️ Common Mistakes
- Downloaded JDK but forgot to add it to IntelliJ
- Confused Java Runtime (JRE) with Java Development Kit (JDK)
- Language level is too low for features used (e.g., using records on Java 8)
Fixing the SDK and language level clears up many Java symbol not found errors and helps IntelliJ recognize your code base correctly.
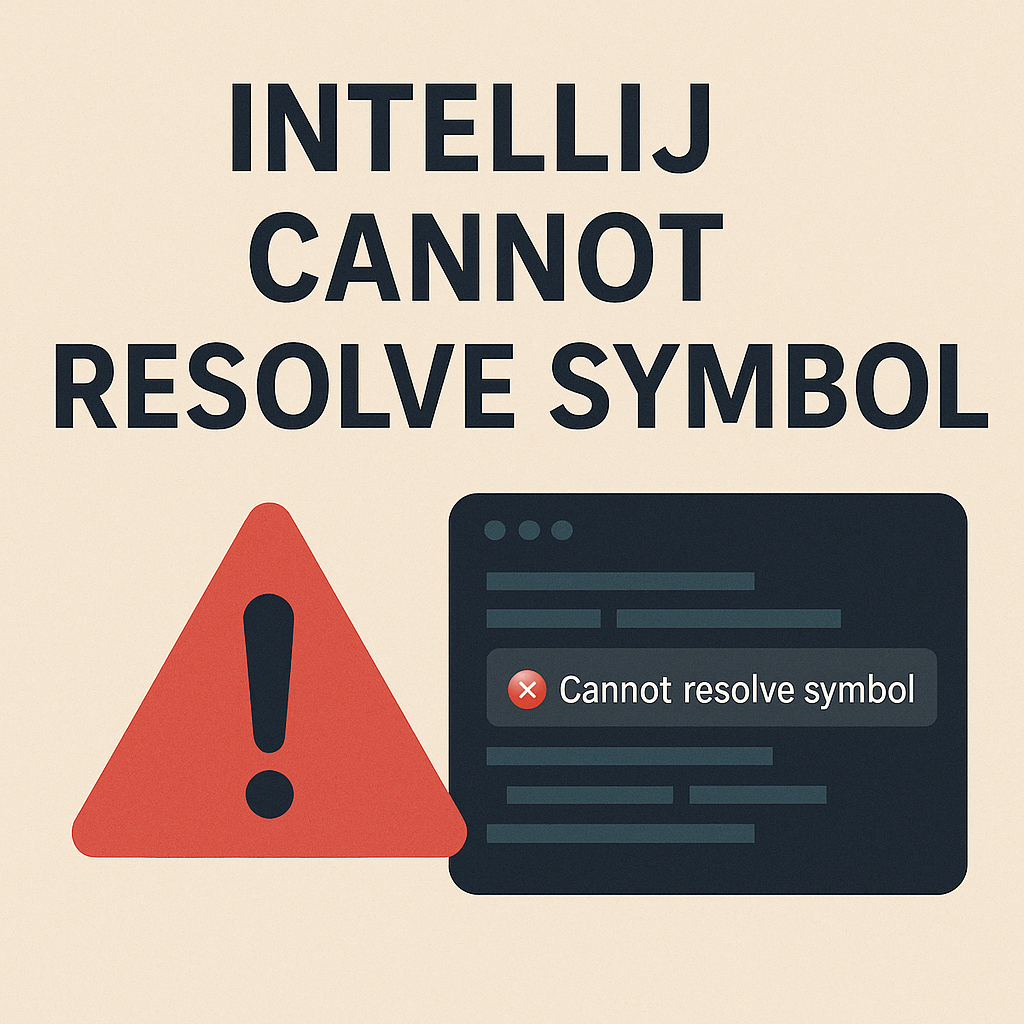
đź§© Section 4: Fix IntelliJ Cannot Resolve Symbol by Verifying Module and Classpath Settings
Even with the correct SDK and synced dependencies, the IntelliJ cannot resolve symbol error can still appear if your module settings or classpath configuration are broken.
🔍 How Module Misconfiguration Triggers Symbol Errors
If IntelliJ doesn’t recognize certain folders as source roots, it won’t index the files within them. As a result, any classes or methods inside those folders may show up as “unresolved.”
This is especially common when:
- Importing projects from GitHub or other machines
- Working with multi-module builds
- Manually creating or moving folders without updating IntelliJ
âś… How to Check and Fix Module Structure
Step-by-step:
- Go to
File > Project Structure > Modules
- Select the relevant module
- Under the “Sources” tab:
- Ensure folders like
src/main/java
andsrc/test/java
are marked correctly:- Blue folder = “Sources”
- Green folder = “Test Sources”
- Ensure folders like
- Under the “Dependencies” tab:
- Make sure required libraries, SDK, or other modules are listed
📦 Fix Classpath Issues
Sometimes external JARs or libraries are not added to the classpath, resulting in missing symbol errors.
To manually add:
- Go to
File > Project Structure > Libraries
- Click the
+
icon to add a missing JAR or library - Rebuild the project
đź§ Why This Works
When IntelliJ doesn’t know where your source code lives—or what libraries it depends on—it fails to resolve references even if the files are right there. Properly configuring the module and classpath helps restore IntelliJ’s ability to resolve symbols.
đź’ˇ Bonus Tip
You can right-click any folder in the Project view and choose:
Mark Directory as > Sources Root
- or
Test Sources Root
Doing this manually is sometimes faster than going through Project Structure settings.
đź—‚ Section 5: Invalidate Caches and Restart IntelliJ to Resolve Symbol Errors
If none of the earlier solutions worked, and you’re still stuck with the IntelliJ cannot resolve symbol error, it’s time to invalidate IntelliJ’s caches. This method solves many deep-rooted issues related to indexing, class resolution, and corrupted project metadata.
🔍 Why Cache Corruption Causes Symbol Resolution Issues
IntelliJ IDEA maintains an internal cache to speed up features like autocomplete, symbol lookup, and refactoring. However, this cache can become out of sync or corrupted during:
- Project imports
- IntelliJ updates
- Git branch switching
- Large refactors or file renaming
When this happens, IntelliJ might fail to recognize symbols that are perfectly valid—leading to persistent symbol not found errors.
âś… How to Invalidate Caches and Restart
- Click on the File menu in IntelliJ
- Choose Invalidate Caches / Restart…
- In the dialog, click Invalidate and Restart
IntelliJ will restart and begin rebuilding the cache from scratch. Once this process is complete, try reopening your project and see if the “IntelliJ cannot resolve symbol” error disappears.
đź§ What This Actually Does
Invalidating caches:
- Clears all existing indexing data
- Removes temporary files
- Forces IntelliJ to re-analyze all project files
- Resets internal project structure metadata
This often fixes the most stubborn IntelliJ resolve issues, especially when other fixes like Gradle sync or SDK tweaks fail.
⚠️ Warning
This operation can take a few minutes to complete, especially for large projects. But it’s safe and won’t delete any code or project files.
đź’ˇ Pro Tip
After restarting, be sure to:
- Clean & Rebuild the Project (next section)
- Verify that SDK and dependencies are still correctly set
This ensures that your fresh cache is built on top of correct configurations.
đź§Ľ Section 6: Clean and Rebuild Your Project to Eliminate IntelliJ Cannot Resolve Symbol Errors
Sometimes, IntelliJ IDEA holds onto outdated compiled files or temporary build artifacts that interfere with symbol recognition. In such cases, the solution is simple but effective: clean and rebuild your project.
This action can resolve lingering IntelliJ cannot resolve symbol errors that weren’t fixed by syncing dependencies or invalidating caches.
🔍 Why Cleaning the Project Helps Fix Symbol Resolution Issues
Even if the code and configuration are correct, IntelliJ might be using:
- Outdated
.class
files - Incomplete or corrupted intermediate builds
- Mismatched bytecode and source mappings
These inconsistencies confuse IntelliJ’s parser and cause it to misidentify valid code as invalid—resulting in Java symbol not found or IntelliJ resolve issue errors.
âś… How to Clean and Rebuild in IntelliJ
- Click
Build
on the top menu - Select
Clean Project
- Once that completes, go to
Build > Rebuild Project
This process will:
- Delete all compiled class files
- Recompile the project from scratch
- Re-index all source files
⚠️ What to Expect
- Clean Project removes all existing build output.
- Rebuild Project recompiles all modules and re-evaluates the classpath.
It’s a safe operation and won’t affect your source files.
đź§ Still Seeing IntelliJ Cannot Resolve Symbol?
If the error persists after a rebuild:
- Double-check for import typos
- Revisit annotation processor settings (next section)
- Consider creating a new project from existing sources as a last resort
đź’ˇ Bonus Tip
Sometimes, toggling between build systems (Gradle ↔ IntelliJ build) can help. You can do this via:
File > Settings > Build, Execution, Deployment > Compiler
- Switch between “Build project automatically” or not, depending on your workflow
đź§ Section 7: Enable Annotation Processing to Fix IntelliJ Cannot Resolve Symbol for Lombok and Others
If you’re using Lombok, MapStruct, or other code-generation libraries, there’s a high chance that IntelliJ IDEA is throwing “IntelliJ cannot resolve symbol” errors for generated methods or constructors. This usually happens because annotation processing is disabled by default in IntelliJ.
🔍 Why Annotation Processors Matter
Libraries like Lombok generate boilerplate code—such as getters, setters, constructors, and builders—at compile time using annotations like @Getter
, @Builder
, and @NoArgsConstructor
.
If annotation processing is turned off:
- IntelliJ won’t see the generated code
- Symbols like
.getX()
ornew Builder()
appear missing - But your project may still compile without errors via Gradle or Maven
This makes it confusing, because IntelliJ cannot resolve symbol, but the compiler can.
âś… How to Enable Annotation Processing in IntelliJ
- Go to
File > Settings
(orPreferences
on macOS) - Navigate to
Build, Execution, Deployment > Compiler > Annotation Processors
- Check the box: Enable annotation processing
- Click Apply, then OK
- Rebuild the project (
Build > Rebuild Project
)
This will allow IntelliJ to recognize all symbols generated by annotation-based tools like Lombok.
📌 Additional Checks for Lombok
- Ensure Lombok is declared in your
build.gradle
orpom.xml
- Add the Lombok IntelliJ plugin:
- Go to
Settings > Plugins
- Search for Lombok
- Install and restart IntelliJ
- Go to
Without the plugin, IntelliJ still won’t fully understand Lombok’s generated code—even with annotation processing enabled.
đź§ What About Other Annotation Tools?
This solution also applies to:
- MapStruct
- Dagger
- AutoValue
- Immutables
If you’re using any of these tools and experiencing the IntelliJ cannot resolve symbol problem, annotation processing is very likely the missing piece.
đź’ˇ Final Tip
After enabling annotation processing, always perform a clean build and let IntelliJ fully reindex the codebase. This ensures that all generated symbols are properly recognized and indexed.
âť“ FAQ: IntelliJ Cannot Resolve Symbol
1. Why does IntelliJ show “Cannot resolve symbol” even though the class exists?
This usually means IntelliJ hasn’t indexed your project correctly or it can’t locate the necessary dependencies. Try syncing Gradle/Maven and rebuilding the project.
2. How do I fix IntelliJ cannot resolve symbol error for standard Java classes like Scanner or List?
Check if the Project SDK is set properly. If IntelliJ doesn’t have access to a valid JDK, it won’t recognize core Java classes.
3. What does “IntelliJ cannot resolve symbol” mean exactly?
It means IntelliJ IDEA cannot find the definition of the symbol in the current project context—even if it exists in the codebase.
4. Will invalidating IntelliJ caches fix symbol resolution errors?
Yes. Invalidating caches often fixes issues where the IDE is out-of-sync with your project. Go to File > Invalidate Caches / Restart
.
5. Does this error affect the actual build or just the IDE?
Usually, the error only affects IntelliJ. If you can compile the project using javac
, Gradle
, or Maven
, then it’s likely an IDE indexing problem.
6. Why can’t IntelliJ resolve Lombok-generated methods like getX()?
Lombok requires both the Lombok plugin and annotation processing to be enabled in IntelliJ. Make sure both are set correctly.
7. How do I know if annotation processing is enabled?
Go to File > Settings > Build, Execution, Deployment > Compiler > Annotation Processors
and check the box for “Enable annotation processing”.
8. Can incorrect import statements trigger IntelliJ cannot resolve symbol?
Yes. Typos or missing import statements can lead to this error. Use Alt + Enter
to auto-import missing classes.
9. How do I fix IntelliJ cannot resolve symbol in multi-module projects?
Check that all modules are correctly added and that dependencies are properly declared in Project Structure > Modules > Dependencies
.
10. Does renaming files or packages cause this error?
Yes. If IntelliJ hasn’t reindexed after a rename or refactor, it might not recognize updated symbols. Try cleaning and rebuilding the project.
11. Can IntelliJ plugins affect symbol resolution?
Absolutely. Certain plugins can interfere with code recognition. Try disabling unnecessary plugins or resetting IntelliJ to default settings.
12. Should I recreate the project if nothing works?
As a last resort, yes. You can create a new project and import the source code again. This often resolves deep-seated IntelliJ configuration issues.
13. Is there a log file to diagnose IntelliJ cannot resolve symbol errors?
Yes. Go to Help > Show Log in Explorer
(or Finder) to access IntelliJ logs. Search for indexing or dependency errors there.
For additional details, refer to the official IntelliJ support article on symbol resolution.